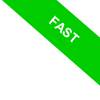
Python Function Annotations
In Python, function annotations offer a way to attach additional metadata to the parameters and return value of a function. This feature enhances code readability and provides valuable insights about function usage.
Purpose of Annotations: Function annotations serve as a powerful aid in making your code more intuitive. They allow you to specify expected data types for parameters and return values, although their use is entirely optional and does not impact program execution. Annotations are incredibly beneficial during development and debugging, offering clear guidance on the intended use of functions.
Python introduced this feature in version 3.0.
Utilizing Annotations:
- Parameter Annotations:
To annotate a parameter, place a colon (:) after the parameter name, followed by the expected data type. If the parameter has a default value, it should follow the type annotation. For example, in 'function(number:int=5)', 'int' is the annotated type for 'number'. - Return Value Annotations:
Function return types can also be annotated. This is done by appending an arrow (->) after the parameters list, followed by the type of the return value, such as 'function(number) -> 'comment''.
Remember, annotations are limited to regular functions and cannot be used in anonymous functions.
Annotations provide guidance rather than enforce type constraints. Therefore, while you can annotate a parameter as a specific type (e.g., 'int'), it doesn’t restrict passing a different type (e.g., 'str'). For actual runtime type checks, consider using external tools like mypy or implement manual checks in your code.
An Example in Practice
Consider this straightforward annotated function:
def greet(name: str, year:int=2000) -> str:
return f"Hello, {name}!"
Here, 'name: str' suggests that 'name' should be a string. The '-> str' annotation signifies that the function will return a string.
Annotations are accessible through the function’s __annotations__ attribute.
print(greet.__annotations__)
{'name': <class 'str'>, 'year': <class 'int'>, 'return': <class 'str'>}
Unlike docstrings, annotations offer a more specific way to document the intended types for parameters and return values.
However, due to Python's dynamic typing, annotations do not influence program execution. For instance, calling 'greet' with a number (5) instead of a string still executes successfully.
print(greet(5))
Hello 5!
To enforce annotated types, run the program with mypy:
mypy programName.py
mypy is an external static type checker that scrutinizes annotated types and detects inconsistencies. It operates from the command line and may increase computational resource usage.
Annotations can also be used for adding descriptive strings, providing additional context about parameters.
def speed(s: 'distance in meters', t:'time in seconds'=1) -> 'speed of an object':
return s/t
Furthermore, you can introspect and query individual parameter annotations.
print(speed.__annotations__['t'])
time in seconds
This functionality allows IDEs to leverage these annotations for explaining the role of each parameter in a function.
In summary, while annotations enrich your code with clarity and maintainability, they don't alter program behavior.