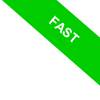
Method and Function Overloading in Python
This guide explores method and function overloading in Python.
What is overloading? Overloading, or method overloading, allows you to define multiple methods with the same name but different parameters within the same class. This flexibility lets you call the same method in different ways depending on the arguments you pass. Overloading can also be applied to functions.
Python does not support traditional method overloading like languages such as Java or C++, but we can achieve similar results through creative approaches.
Specifically, there are techniques that allow you to achieve a similar effect. We'll explore two alternative approaches: using default arguments and variable arguments.
Using Default and Variable Arguments
One way to simulate overloading in Python is by using default and variable arguments.
This allows you to call a method with a variable number of arguments and handle different situations with a single method or function.
Function Example
For instance, let's say you want to write a function that can add two or three numbers. You could define it like this:
def sum(a, b, c=None):
if c is None:
return a + b
else:
return a + b + c
Depending on whether you pass two or three arguments, the function performs different calculations.
print(sum(2, 3))
5
If you pass three arguments:
print(sum(2, 3, 4))
9
Here, the optional parameter `c` defaults to `None` if not specified, and the function acts as a simple sum of two numbers.
Class Method Example
You can apply the same approach to a class method since a method is a local function within a class.
For example, create a `Calculator` class with a `sum` method that can add two or three numbers based on the provided arguments.
class Calculator:
def sum(self, a, b, c=None):
if c is None:
return a + b
else:
return a + b + c
Then create an instance of the `Calculator` class:
calc = Calculator()
Now call the sum() method with two arguments:
print(calc.sum(2, 3))
5
And call the same method with three arguments:
print(calc.sum(2, 3, 4))
9
As you can see, you can call the `sum()` method of the `Calculator` class with either two or three numeric arguments. If there are two arguments, the method sums them. If you provide a third argument, the method sums all three.
Using Variable Arguments
Another more flexible approach is using variable arguments, which allow you to pass an arbitrary number of arguments to the method.
For instance, create a `Calculator` class:
class Calculator:
def sum(self, *numbers):
"""Sums an arbitrary number of arguments."""
return sum(numbers)
In this case, the `sum()` method in the `Calculator` class can accept a variable number of arguments thanks to the `*numbers` parameter, which collects all passed arguments into a tuple.
The method then uses the `sum()` function to add all elements of the tuple.
Let's test it by creating an instance of the `Calculator` class:
calc = Calculator()
Call the sum() method with two arguments:
print(calc.sum(2, 3))
5
Then with three arguments:
print(calc.sum(2, 3, 4))
9
And finally, with five arguments:
print(calc.sum(1, 2, 3, 4, 5))
15
This way, you can use the same method to sum an indefinite number of arguments.
Using the `functools` Module
Another approach to achieving overloading effects is using decorators from the functools module.
This allows you to create overloadable functions or methods based on the type of one or more arguments.
Function Example
Suppose you want to write a function that handles different input types differently.
You can use the @singledispatch decorator to define a base function and then add specific behaviors for different data types.
from functools import singledispatch
@singledispatch
def describe(data_type):
print(f"Generic type: {type(data_type)}")
@describe.register(int)
def _(data_type):
print(f"Integer: {data_type}")
@describe.register(list)
def _(data_type):
print(f"List with {len(data_type)} elements")
When you call the describe() function, it behaves differently depending on the type of data you pass as an argument.
If you pass an integer:
describe(42)
Integer: 42
If you pass a list:
describe([1, 2, 3, 4])
List with 4 elements
Essentially, the `describe()` function adapts its behavior based on whether it receives an integer or a list.
You can add more specific behaviors as needed.
How to Associate Multiple Types with a Function Argument?
To link multiple types to an argument, you need to apply multiple decorators to the function sequentially.
For instance, to handle both 'float' and 'int' types, you should write:
@descrivi.register(float)
@descrivi.register(int)
def _(type):
print(f"The number is {type}")
Keep in mind that the @singledispatch decorator only affects the first argument of the function.
How to Call an Existing Function Based on an Argument
In Python, you can link an argument's data type to an existing function.
For example, if the argument of the descrivi()
function is a string, it will call the foo()
function.
from functools import singledispatch
@singledispatch
def descrivi(tipo):
print(f"The generic type is {type(tipo)}")
@descrivi.register(int)
def _(arg1):
print(f"The integer is {arg1}")
def foo(arg1):
print('The string is', arg1)
descrivi.register(str, foo)
Therefore, if you call the descrivi()
function with an integer, it will respond as follows:
descrivi(1)
The integer is 1
If you call the descrivi()
function with a string, it will call the foo()
function, and the result will be:
descrivi('hello')
The string is hello
Class Method Example
The `singledispatch` decorator is designed for module-level functions and cannot be used directly with class methods.
However, you can work around this limitation by using the @singledispatchmethod decorator.
The @singledispatchmethod decorator is a version of @singledispatch designed to be used with class methods. It allows you to define a base method that is then specialized for different data types through additional registered methods.
For example, let's say you have a class that handles various input types for a specific operation.
In this case, create a `Calculator` class with a `describe` method that behaves differently depending on the type of data provided.
from functools import singledispatchmethod
class Calculator:
@singledispatchmethod
def describe(self, data):
raise NotImplementedError("Not supported")
@describe.register(int)
def _describe_int(self, data):
return f"Processed integer: {data}"
@describe.register(str)
def _describe_str(self, data):
return f"Processed string: {data.upper()}"
@describe.register(list)
def _describe_list(self, data):
return f"Processed list with {len(data)} elements"
Now create an instance of the `Calculator` class:
proc = Calculator()
Call the `describe()` method with different input types, such as an integer:
print(proc.describe(123))
Processed integer: 123
Then call the method with a string argument:
print(proc.describe("hello"))
Processed string: HELLO
Finally, call the method with a list:
print(proc.describe([1, 2, 3, 4]))
Processed list with 4 elements
The use of `singledispatchmethod` helps you manage multiple data types within the same class, providing specific implementations for each type.
Overloading Arithmetic Operations
Python also allows you to define custom behavior for arithmetic operators within class objects.
In other words, you can specify how arithmetic operators (like +, -, *, etc.) should behave when used with instances of a custom class.
For instance, let's create a `Vector2D` class that represents a two-dimensional vector.
class Vector2D:
def __init__(self, x, y):
self.x = x
self.y = y
Now create two objects of this class:
v1 = Vector2D(2, 3)
v2 = Vector2D(4, 5)
You cannot add these objects using the addition operator '+':
v3 = v1 + v2
TypeError: unsupported operand type(s) for +: 'Vector2D' and 'Vector2D'
To overcome this issue, you can override the behavior of the '+' operator within the class using the __add__ method.
class Vector2D:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
return Vector2D(self.x + other.x, self.y + other.y)
Now create new instances:
v1 = Vector2D(2, 3)
v2 = Vector2D(4, 5)
When you try to add them now, Python executes the __add__ method when it encounters the '+' operator, returning the vector sum:
v3 = v1 + v2
The result is another `Vector2D` object with values (6, 8).
To explain what happened: the sum of two vectors is obtained by adding their respective x and y components.
You can access the individual components by accessing the 'x' and 'y' attributes:
print(v3.x, v3.y)
6 8
This way, you've overridden the behavior of the '+' operator with the '__add__' method, but only within the `Vector2D` class objects.
Overloading arithmetic operators allows you to use intuitive and natural syntax for complex operations.
In general, you can use the same approach to add, subtract, and calculate the dot product of two vectors using the `+`, `-`, and `*` operators.
class Vector2D:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
return Vector2D(self.x + other.x, self.y + other.y)
def __sub__(self, other):
return Vector2D(self.x - other.x, self.y - other.y)
def __mul__(self, other):
return self.x * other.x + self.y * other.y # Dot product
def __repr__(self):
return f"Vector2D({self.x}, {self.y})"
By writing `v1 - v2`, Python calls `v1.__sub__(v2)`, creating a new vector with components equal to the difference of the original vectors' components.
By writing `v1 * v2`, Python calls `v1.__mul__(v2)`, calculating the dot product of the two vectors.
Finally, the `__repr__` method specifies how Python should represent the object when it is displayed. For example, when you execute `print(v3)`, it returns the string "Vector2D(1, 2)" instead of the object's memory reference.
Commutative Operations
When overloading arithmetic operations, it's crucial to remember that these methods aren't inherently commutative.
For example, consider the scenario of multiplying a vector by a scalar.
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __mul__(self, scalar):
if isinstance(scalar, (int, float)):
return Vector(self.x * scalar, self.y * scalar)
return
def __repr__(self):
return f"Vector({self.x}, {self.y})"
Overloading the "__mul__()" method allows you to use the * operator to multiply a vector by a number.
v = Vector(1, 2)
print(v * 3)
Python identifies that the first operand is a vector and the second is a scalar, so it invokes the overloaded __mul__()
method and returns the result.
As a result, the operation v*3 produces the vector Vector(3,6), which is the original vector Vector(1,2) with its x and y components multiplied by three.
Vector(3, 6)
However, if you try to multiply a scalar by a vector, Python won't call the __mul__()
method because the first operand is a scalar, not a vector.
In this case, the operation 3*v will raise an error.
v = Vector(1, 2)
print(3 * v)
Traceback (most recent call last):
TypeError: unsupported operand type(s) for *: 'int' and 'Vector'
To make the multiplication commutative, you need to implement the "__rmul__()" method, which handles the operation when the scalar comes first.
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __mul__(self, scalar):
if isinstance(scalar, (int, float)):
return Vector(self.x * scalar, self.y * scalar)
return
def __rmul__(self, scalar):
# Leverage the same method to make multiplication commutative
return self.__mul__(scalar)
def __repr__(self):
return f"Vector({self.x}, {self.y})"
Now, whether you multiply a vector by a scalar or a scalar by a vector, the operation works seamlessly.
v = Vector(1, 2)
print(v * 3)
print(3 * v)
Vector(3, 6)
Vector(3, 6)
In a similar fashion, you can make addition commutative by implementing the "__radd__()" method.
Dispatch Attribute
The dispatch attribute allows you to call the function associated with a specific type.
For instance, consider the following function:
from functools import singledispatch
@singledispatch
def describe(obj):
print(f"The argument type is {type(obj)}")
@describe.register(int)
def _(arg1):
print(f"The integer is {arg1}")
@describe.register(str)
def _(arg1):
print(f"The string is {arg1}")
The describe() function behaves differently depending on whether the argument is an integer (int) or a string (str).
If you want to call the function associated with integers (int), regardless of the argument type, you can use the dispatch attribute.
fun = describe.dispatch(int)
Alternatively, you can use the registry attribute, which stores all the type-to-function mappings. The result is the same.
fun=descrivi.registry[int]
Now, call the function fun() passing a string as the argument:
fun("one")
Even though the argument is a string ("one"), the dispatch attribute calls the function associated with integers.
The integer is one.
Note that if the dispatch() attribute doesn't find the associated type, it will call the main implementation associated with the object.
fun = describe.dispatch(float)
fun("one")
The argument type is <class 'str'>
This is another useful feature of the Python language that is worth knowing.