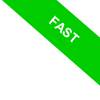
Python Subgenerators
In Python, a subgenerator is essentially a generator function that is embedded within another generator to yield a sequence of values.
Purpose of Subgenerators: The primary advantage of using subgenerators lies in their ability to simplify the structure of code that performs intricate iteration tasks. This technique allows for the modularization of code, especially useful in managing complex iteration processes. By breaking down the logic into smaller, more manageable parts, subgenerators promote code reusability and maintainability.
Defining a subgenerator is straightforward: you write a function that employs the yield statement to produce values incrementally.
This function can be invoked within another generator function, enhancing the modularity of your code.
Subgenerators often leverage the yield from statement, which allows for the delegation of iteration work to the subgenerator, streamlining the flow of values.
yield from subgenerator
Employing both a main generator and a subgenerator facilitates the sequential processing of values, minimizing memory usage compared to traditional iterative methods.
An Illustrative Example
Below is a straightforward example demonstrating how a subgenerator can be effectively utilized within a primary generator.
# Generator that yields numbers from 1 to n
def generate_numbers(n):
for num in range(1, n + 1):
yield num
# Generator for finding and yielding a given number's divisors
def find_divisors(num):
for i in range(1, num + 1):
if num % i == 0:
yield i
# Main generator employs 'yield from' to directly pass on divisors of each number
def numbers_and_divisors(n):
for num in generate_numbers(n):
print(f"Divisors of {num}: ", end="")
# 'yield from' is used here to seamlessly yield values from the subgenerator
yield from find_divisors(num)
print()
# Utilizing the setup with n=10
for divisor in numbers_and_divisors(10):
print(divisor, end=" ")
The workflow of this program is as follows:
For each number from 1 to n, the main generator, numbers_and_divisors, announces the number and initiates the search for its divisors.
It's important to note that the series of numbers from 1 to n is generated by another generator, generate_number, which is invoked by the main generator within a loop.
Next, numbers_and_divisors employs yield from to effortlessly transfer control to the subgenerator, find_divisors, which sequentially identifies and yields each divisor back to the main generator.
Once all divisors for a number are yielded, the loop in the main generator progresses to the next number, continuing until the entire range from 1 to n is processed.
In this instance, with n=10, the script outputs the divisors for numbers from 1 to 10.
Divisors of 1: 1
Divisors of 2: 1 2
Divisors of 3: 1 3
Divisors of 4: 1 2 4
Divisors of 5: 1 5
Divisors of 6: 1 2 3 6
Divisors of 7: 1 7
Divisors of 8: 1 2 4 8
Divisors of 9: 1 3 9
Divisors of 10: 1 2 5 10
This demonstration aims to clarify the operation and significant advantages of subgenerators within Python programming, offering a streamlined and memory-efficient approach to handling extensive sets of data.
Imagine the computational and memory resources required to calculate divisors for numbers ranging up to a billion using traditional iterative approaches. Generators and subgenerators, by yielding values incrementally, dramatically reduce the memory footprint, making your programs more efficient and scalable.