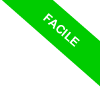
Python Function Introspection
In programming, introspection is the capability of a program to examine its own type or the properties of objects during runtime. Python's introspection is particularly invaluable for a deeper understanding and exploration of how functions operate.
What Exactly is Function Introspection? In the context of Python, introspection involves accessing information about a function as the program is running. This includes, but is not limited to, the function's name, the parameters it accepts, its documentation (docstring), and more.
There are numerous methods to get an inside look at a function. This tutorial will cover the primary approaches.
Inspecting Object Attributes
One common approach for introspection is the use of object attributes.
Take, for instance, creating a function:
def example(param1, param2=2):
"""This function serves as an example."""
return param1 + param2
To discover the name of the function, you can employ the __name__ attribute.
print(example.__name__)
example
If you wish to view the docstring, the __doc__ attribute is your go-to.
print(example.__doc__)
This function serves as an example.
These instances are just the tip of the iceberg. There are many other attributes to explore.
For example, the __defaults__ attribute reveals the default values of a function.
print(example.__defaults__)
(2,0).
The __code__ attribute, for instance, includes a wealth of information about the function.
info=example.__code__
# Argument count
print(info.co_argcount)
# Variable names
print(info.co_varnames)
# Local variables count
print(info.co_nlocals)
# Keyword-only argument count
print(info.co_kwonlyargcount)
# Constants used
print(info.co_consts)
2
('param1', 'param2')
2
0
('This function serves as an example.',)
The Power of the inspect Library
The "inspect" library stands as a cornerstone for introspective activities in Python.
This module equips you with targeted functions to glean real-time details about objects, including functions.
For instance, start by creating a function:
def example(param1, param2):
"""This function serves as an example."""
return param1 + param2
Then, bring the inspect module into play.
import inspect
The inspect.signature() method is your key to unlocking the parameters of a function.
parameters = inspect.signature(example)
print(parameters)
(param1, param2)
This code snippet effortlessly brings all the parameter information of the function to your fingertips.
If you're also interested in the type of parameters (e.g., positional, keyword, etc.), the "parameters" attribute is incredibly useful.
parameters = inspect.signature(example).parameters
for name, parameter in parameters.items():
print(f"{name}: {parameter.kind}")
param1: POSITIONAL_OR_KEYWORD
param2: POSITIONAL_OR_KEYWORD
This code offers a clear view of both the parameter names and their types.
Further Insights with inspect
The inspect module extends its utility with methods like ismodule(), ismethod(), isfunction(), iscode(), and isbuiltin(), each serving to ascertain if an object fits a specific category - be it a module, method, function, code, or a built-in object.
inspect.isfunction(example)
True
For a deep dive into the documentation, the getdoc() method retrieves both the docstring and comments.
inspect.getdoc(example)
This function serves as an example.
The getsource() method is another gem, revealing the source code of the object. And there's more where that came from.
Dynamic Introspection with inspect
Dynamic introspection with the inspect module opens up a world of possibilities. It allows you to adapt your actions based on the parameter types a function accepts.
For example:
import inspect
def execute_with_introspection(function, *args):
if 'param2' in inspect.signature(function).parameters:
return function(*args)
else:
return "This function lacks the parameter 'param2'"
Let's apply it to the function example() with parameters 5 and 10:
result = execute_with_introspection(example, 5, 10)
print(result)
Here, "execute_with_introspection" checks for the presence of a 'param2' parameter before running the function. Since 'param2' exists, example(5,10) is successfully executed.
15
This is just a glimpse into the vast potential of introspection in Python.
As you delve deeper, you'll uncover even more powerful and versatile ways to leverage this functionality.
Exploring Functions with the dis Library
The "dis" module offers yet another perspective on function introspection.
Start by crafting a function:
def example(a, b=2, *varargs, c=99, **kwargs):
"""This function serves as an example."""
return a + b
Next, import the dis module and utilize the show_code() function.
import dis
info=dis.show_code(example)
print(info)
With this singular command, you gain access to a comprehensive overview of the function.
Name: example
Filename: /home/main.py
Argument count: 2
Positional-only arguments: 0
Kw-only arguments: 1
Number of locals:& nbsp; 5
Stack size: 2
Flags: OPTIMIZED, NEWLOCALS, VARARGS, VARKEYWORDS, NOFREE
Constants:
0: 'This function serves as an example.'
Variable names:
0: a
1: b
2: c
3: varargs
4: kwargs
None
These tools are just a few examples of the many ways to explore the intricacies of functions through introspection.