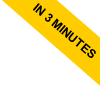
Context Manager in Python
A context manager is a construct that allows you to manage resources automatically by implementing two special methods: `__enter__()` and `__exit__()`.
- `__enter__(self)`
This method is executed at the start of the `with` block. It is used to acquire the necessary resources and can return a value that will be assigned to the variable following `as`. - `__exit__(self, exc_type, exc_val, exc_tb)`
This method runs at the end of the `with` block, whether it exits normally or due to an exception. It is used to release the acquired resources.The parameters `exc_type`, `exc_val`, and `exc_tb` provide information about any exception that might have been raised. If the method returns `True`, the exception is suppressed.
These methods are called at the beginning and end of the context by the `with` statement.
You can define a context manager using either classes or functions.
What are context managers used for? They are very useful for handling resources that need to be acquired and released, such as files, network connections, or synchronization blocks. Context managers ensure that resources are always released correctly, regardless of how the associated block of code exits.
An Example
Here is an example of a context manager implemented as a class using the with statement:
class MyContextManager:
def __enter__(self):
print("Entering the context")
# Code to acquire the resource
return self
def __exit__(self, exc_type, exc_val, exc_tb):
print("Exiting the context")
# Code to release the resource
if exc_type:
print(f"An exception occurred: {exc_val}")
return True # Return True to suppress the exception, False otherwise
with MyContextManager() as manager:
# Code within the context
print("Inside the context")
In this example, we defined the class 'MyContextManager()' with the methods __enter__ and __exit__.
When we call the class with the with statement, the __enter__ method is executed first, followed by the code inside the context, and finally, the __exit__ method.
This is the program's output:
Entering the context
Inside the context
Exiting the context
You can also create context managers using functions
Here is another example of a context manager created using a function.
In this case, we created a context manager by decorating the function with @contextmanager
from contextlib import contextmanager
@contextmanager
def my_context_manager():
print("Entering the context")
try:
yield
print("Inside the context")
finally:
print("Exiting the context")
with my_context_manager():
# Code within the context
print("Doing something inside the context")
In this case, the __enter__ method executes the code before the yield statement.
The __exit__ method, on the other hand, executes the code following the yield statement.
The output is as follows:
Entering the context
Doing something inside the context
Inside the context
Exiting the context
Note that in case of an error, the __exit__ method is not executed, but the finally clause is always executed.
Therefore, if you want the __exit__ method to always be executed, you should place it within the finally clause.