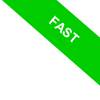
Variadic Functions in Python
Variadic functions in Python are a flexible tool, allowing for the receipt of a variable number of arguments. This adaptability is key in many programming scenarios.
Purpose and Utility: These functions shine when the exact number of inputs is not predetermined. Python supports two varieties: those accepting variadic positional arguments, and those handling key-value pairs.
Variadic Positional Arguments
Such functions employ an asterisk (*) before the parameter name, effectively collating additional positional arguments into a tuple.
- def positional_variadic_function(*args):
- for arg in args:
- print(arg)
Here, args is the tuple holding all passed arguments. For example, calling the function with three parameters:
positional_variadic_function(5, 2020, "London")
This results in a tuple receiving the three parameters.
5
2020
London
In this tuple, args[0] contains the first parameter (5), args[1] the second (2020), and args[2] the third ("London").
This capability allows the function to handle an undefined number of parameters.
Customizable Naming: While "args" is a conventional name, it's not a requirement. Any name can follow the asterisk, like *parameters or *list, adapting to your coding style.
Trivia: The naming convention of "args" or "varargs" in Python draws inspiration from the C language's "varargs.h" header file, instrumental in managing variable argument lists in C programs.
Variadic Key-Value Arguments
Functions designed for key-value pairs use a double asterisk (**) before the parameter name, capturing arguments in a dictionary.
- def key_value_variadic_function(**kwargs):
- for key, value in kwargs.items():
- print(f"{key}: {value}")
In this instance, kwargs is the dictionary containing all key-value pairs. For example:
key_value_variadic_function(year=2020, month=5, country="USA")
Here, the function neatly organizes these pairs into the kwargs dictionary.
year: 2020
month: 5
country: USA
This feature proves invaluable when dealing with functions where parameters might vary extensively.
For instance, it's particularly effective for configuring objects with numerous default-value parameters.
Mixing Argument Types in Variadic Functions
Combining normal arguments with *args and **kwargs in a single function is possible, respecting the order: first normal parameters, then *args, followed by **kwargs.
function(normal parameters, *args, **kwargs)
A practical example clarifies this concept:
- def mixed_arguments_function(name, *args, **kwargs):
- print(f"Name: {name}")
- # Processing positional arguments
- for arg in args:
- print(f"Positional Argument: {arg}")
- # Handling key-value pairs
- for key, value in kwargs.items():
- print(f"{key}: {value}")
In this scenario, "name" is a standard argument, while *args captures additional positional arguments (like 1, 2, 3) into a tuple. The **kwargs parameter rounds up key-value arguments (like city="Rome", age=30) into a dictionary.
When the function is called with multiple arguments:
mixed_arguments_function("Mario", 1, 2, 3, city="Rome", age=30)
"Mario" is stored as "name," the positional arguments in args, and the key-value pairs in kwargs.
The output is:
Name: Mario
Positional Argument: 1
Positional Argument: 2
Positional Argument: 3
city: Rome
age: 30
This flexibility allows for the creation of highly adaptable functions, accommodating a wide range of parameters and argument types.