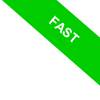
Iterable Unpacking in Python Functions
In this lesson, we're going to delve into a sophisticated technique in Python that elegantly combines iterable unpacking with function parameter matching.
Prerequisites: A fundamental grasp of Python is essential before exploring these advanced concepts. Familiarity with functions, lists, tuples, and loops is recommended.
Understanding Unpacking
Iterable unpacking, or simply unpacking, is a powerful feature in Python. It allows for the assignment of elements from an iterable, like lists or tuples, to individual variables.
a, b, c = [1, 2, 3]
Here, "a" is assigned the value 1, "b" is 2, and "c" is 3. It’s straightforward and efficient.
This concept isn’t just limited to variable assignment. It’s incredibly useful in function calls, where you can unpack a list or tuple, assigning each element to a separate function argument.
Let's consider a real-world example:
- def sum(a, b, c):
- return a + b + c
- numbers = [1, 2, 3]
- print(sum(*numbers))
The function takes an iterable, unpacks it into parameters a, b, and c, and then computes the sum. The elegance of this approach lies in its simplicity and clarity.
The output, unsurprisingly, is 6.
6
It’s crucial, however, to ensure that the iterable's length corresponds with the number of parameters in the function. If they don’t match, Python raises an error.
For more flexible scenarios, Python's asterisk "*" symbol comes into play. It allows you to pass a variable number of arguments to a variadic function.
- def print_everything(*args):
- for item in args:
- print(item)
- print_everything(*[1, 2, 3, 4])
Here, the iterable can contain any number of elements, all of which are passed to the function and stored in the tuple "args".
The function iterates over this tuple and prints each element, demonstrating Python's flexibility and power.
1
2
3
4
This method provides a versatile approach to parameter handling in functions.
I hope this guide proves insightful and enhances your Python programming skills. Should you require further information or examples, don't hesitate to reach out.