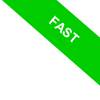
Anonymous Functions in Python
In Python, anonymous functions, also referred to as "lambda functions," are unique in that they don't bear a name.
Notable for their conciseness, these functions stand out due to their unnamed nature.
They are particularly useful for temporary, straightforward tasks requiring a minimalistic approach.
Why Use Them? They shine when a function is needed temporarily and crafting a full function definition seems overkill. Lambda functions offer a swift and streamlined way to write minor functions. Additionally, they're a boon in functional programming, where functions often serve as arguments to other functions.
Defining a lambda function in Python goes like this:
lambda arguments: expression
Breaking it down:
- lambda is the initiating keyword. Unlike the "def" statement, it's an expression, making it versatile for use within other expressions.
- arguments resemble those in standard functions and are what you pass to the function.
- expression refers to the computation performed and its result returned by the function.
By not being bound to a label, the "lambda" keyword effectively creates an anonymous function.
A key characteristic of lambda functions is their ability to accept multiple arguments while being restricted to a single expression.
Practical Examples
Let's delve into some real-world examples.
Consider creating a function that doubles its input value. In a standard function, it looks like this:
- def double(x):
- return x * 2
- print(double(5))
The same can be elegantly achieved with a lambda function:
- double = lambda x: x * 2
- print(double(5))
Here, the anonymous function brings the same result but in a more succinct package.
10
Lambda functions can also handle multiple arguments. For instance, a function that sums two numbers:
- sum = lambda x, y: x + y
- print(sum(5, 3))
8
As demonstrated, lambda functions enhance code compactness and readability.
However, it's wise to avoid overusing them, as they can obscure code readability when used excessively.
A piece of advice: For more intricate functions, the traditional def statement is preferred to maintain clarity.
Integrating with map(), filter(), sorted()
Lambda functions often team up with map(), filter(), and sorted() in list and iterable manipulations.
- map() applies a function across all elements of a list.
- filter() forms a list from elements that a function evaluates to True.
- sorted() arranges list elements in a specified order.
For instance, using map() to square every number in a list:
- numbers = [1, 2, 3, 4, 5]
- squares = map(lambda x: x**2, numbers)
- print(list(squares))
Here, lambda x: x**2 is a succinct function that squares a number. The map() function then applies it to each list item.
The result:
[1, 4, 9, 16, 25]
This method squared each list element without the need for a dedicated function.
Second Example
Consider filtering out even numbers from a list:
Using filter() and a lambda function:
- numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
- even_numbers = filter(lambda x: x % 2 == 0, numbers)
- print(list(even_numbers))
The lambda function lambda x: x % 2 == 0 checks for even numbers. The filter() function then applies it across the list.
The output includes only the even numbers:
[2, 4, 6, 8, 10]
Third Example
Sorting a list of tuples based on the second element, say fruit names:
- list = [(1, 'apple'), (3, 'banana'), (2, 'orange')]
- sorted_list = sorted(list, key=lambda x: x[1])
- print(sorted_list)
The lambda function lambda x: x[1] guides the sorting based on each tuple's second element.
With the sorted() function, the list is rearranged alphabetically by fruit name.
[(2, 'orange'), (3, 'banana'), (1, 'apple')]
This approach is not only potent but also adaptable, letting you sort lists based on nearly any criteria you can express in a single line.