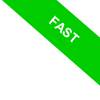
Python's iter() Method
The __iter__() method in Python is a real game-changer. It lets you tag any object as an iterator, opening a whole new world of possibilities.
object.__iter__()
Just by calling object.__iter__(), you get your hands on an iterator object.
But that's just the beginning. Once your iterator object is set up and ready to roll, you can sift through the object's elements from top to bottom. All it takes is the __next__() method.
Here's what happens behind the scenes. The __next__() method retrieves the subsequent value from the iterator. But what if there's nothing left to fetch? That's when it steps up and raises the StopIteration exception.
Now let's break it down with a real-life example, demonstrating how you can implement an iterator in Python, step by step.
First off, we'll need a list. Let's keep it simple and go with three items.
>>> myList=[1,2,3]
Next, we're going to transform this list into an iterator object. The magic spell? You've guessed it: the __iter__() method.
>>> obj=myList.__iter__()
With our iterator set up, we can now take a leisurely stroll through the list items, one by one, simply by calling the __next__() method.
>>>obj.__next__()
1
>>>obj.__next__()
2
>>>obj.__next__()
3
What happens when we've reached the end of the line? Let's see what happens if we dare to invoke the __next__() method again, after the last item of our iterable object.
>>>obj.__next__()
Traceback (most recent call last):
File "/home/main.py", line 6, in <module>
print(obj.__next__())
StopIteration
As predicted, Python raises the StopIteration exception, its subtle way of saying, "Sorry, but there are no more items left to iterate over."
And before we wrap things up, just remember: Python also offers a built-in iter() function, another nifty tool you can use to transform any iterable object into an iterator. Don't forget to give it a spin!