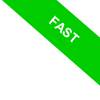
Python's map() Function
In the vast toolkit of Python's built-in functions, map() stands out as a powerful utility. It's designed to apply a specific function to every item within an iterable, such as a list or tuple, and in return, it provides an iterator. Here's the basic structure:
map(function, iterable, ...)
Breaking it down:
- function
This is the operation you wish to apply to each item. - iterable
The collection of items you're working with.
For each iteration, the iterator fetches items from the iterable, processes them through the function, and then yields the result.
So, why should you consider using map()? It's a streamlined way to transform data without the overhead of writing a loop. Plus, since it returns an iterator, memory allocation for the processed items is deferred, making it a memory-efficient choice.
Let's dive into a practical example.
Suppose you have a list of numbers:
list1 = [2,3,4,5]
You can swiftly create an iterator using the bin function, which translates numbers to binary:
z = map(bin, list1)
On invoking the iterator z with the next() function, it processes the list's first item.
next(z)
This action translates bin(2), producing the binary number 0b10.
0b10
Invoke the iterator again:
next(z)
This time, it processes bin(3), resulting in 0b11.
0b11
But map() isn't limited to built-in functions. You can pair it with custom lambda functions too.
Say you have a list of numbers and your goal is to double each:
numbers = [1, 2, 3, 4]
doubled = map(lambda x: x*2, numbers)
You'll get:
print(list(doubled))
Il risultato è una lista con tutti i numeri raddoppiati.
[2, 4, 6, 8]
Moreover, map()
can juggle more than one iterable. In such scenarios, ensure your function can accommodate the number of arguments you're passing.
For instance, with two lists "a" and "b":
a = [1, 2, 3]
b = [10, 20, 30]
You can craft an iterator using a lambda function.
z = map(lambda x, y: x + y, a, b)
Loop through to display the combined results.
- for i in z:
- print(i)
This produces:
11
22
33
For a deeper dive, consider this example.
Define a function, product()
- def product(x,y):
- return x*y
Loop through the results:
- for i in map(product, (2,3,4), (5,6)):
- print(i)
The output? A neat:
10
18
Remember, the loop wraps up once the shorter list (in this case, (5,6)) is exhausted.
While map()
is a gem, don't forget about list comprehension.
For instance, the doubling example can be elegantly written as:
numbers = [1, 2, 3, 4]
doubled = [x*2 for x in numbers]
print(doubled)
And you'll still get:
[2,4,6,8]
Many seasoned developers lean towards list comprehension for its readability. However, the choice between the two often boils down to the specific use case and personal coding style.