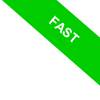
Python's zip() Function
In Python, when you're faced with the task of merging data from multiple sources, the zip() function is your go-to tool. It's designed to pair elements from two or more iterable objects, such as lists, tuples, and dictionaries, producing an iterator of tuples. Consider this basic structure:
zip(*iterables)
Here, *iterables represent two or more iterable objects separated by commas.
The beauty of zip() lies in its simplicity: it yields tuples where each tuple contains elements from all the iterables at the corresponding index.
This function comes in handy when you need to merge related data from different sources.
Let's illustrate with a hands-on example.
Imagine you have two lists, each with three items:
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
Now, create a new iterable by pairing the elements of these two lists using the zip() function:
z = zip(list1, list2)
This new object is an iterator.
So, it includes the __iter__ method
hasattr(z, '__iter__')
True
And the __next__ method
hasattr(z, '__next__')
True
This functionality allows you to sequentially access its items using the next() method.
For instance, calling next(z) the first time gives you the first tuple:
next(z)
(1, 'a')
Each tuple is a pairing of the i-th elements from both lists. Invoke next(z) again, and you'll retrieve:
next(z)
(2, 'b')
One key aspect to remember is that the zip() function is bound by the shortest iterable.
If the iterables have different lengths, zip() will halt once the shortest one is exhausted.
To illustrate, let's work with two lists of unequal lengths:
list1 = [1, 2, 3, 4]
list2 = ['a', 'b', 'c']
The first list has 4 items, while the second has 3.
Now, create an iterator using the zip function:
z=zip(list1, list2)
After zipping and converting the iterator to a list:
list(z)
Notice how the fourth item from list1 is omitted.
[(1, 'a'), (2, 'b'), (3, 'c')]
But what if you're working with more than two iterables? No problem. The zip() function gracefully scales. For three lists:
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
list3 = ['A', 'B', 'C']
Combine these iterables into an iterator.
z=zip(list1,list2,list3)
In this case, the iterator consists of three tuples, each containing three items:
[(1, 'a', 'A'), (2, 'b', 'B'), (3, 'c', 'C')]
To extract the individual iterables from the iterator, first convert the iterator to a list:
y=list(z)
To revert to the original lists, you can cleverly use the zip() function again:
l1, l2, l3 = zip(*y)
This way, you retrieve the content of the original lists:
l1
(1, 2, 3)
In essence, the zip() function is a cornerstone in Python for anyone working with multiple data sequences. It's both powerful and intuitive, making data manipulation a breeze.