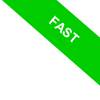
Creating an Iterator Class in Python
Building a class that generates iterator objects allows you to define custom sequences in a flexible way.
The key concept behind an iterator class is to define the behavior of an object that maintains its state during iteration and implements both the `__iter__()` and `__next__()` methods.
Let me give you a practical example.
Imagine you want to create an iterator that generates even numbers up to a specified maximum value.
class EvenNumbersIterator:
def __init__(self, max_value):
self.max_value = max_value # Sets the maximum value for the even numbers to generate
self.current = 0 # Initializes the counter at the first even number
def __iter__(self):
return self # Returns the iterator object itself
def __next__(self):
if self.current > self.max_value:
raise StopIteration # Stops the iteration when the maximum value is exceeded
else:
even_number = self.current
self.current += 2 # Increments to get the next even number
return even_number # Returns the current even number
The `__next__()` method must always include a termination condition, where it raises a `StopIteration` exception.
This is crucial to prevent infinite loops and to signal to a `for` loop that the iteration is complete.
Once the class is defined, you can create an instance of the iterator.
even_numbers = EvenNumbersIterator(10)
Since 'even_numbers' is an iterator object, you can retrieve individual values in sequence by using the `next()` function.
next(even_numbers)
2
next(even_numbers)
4
Each time you call 'next()', the next value in the sequence is returned.
Alternatively, you can use the object you’ve just created in a `for` loop:
for number in even_numbers:
print(number)
The output will be:
0
2
4
6
8
10
You can extend the iterator's functionality to include other features, such as iterating in reverse, skipping specific numbers, or iterating over elements of a sequence based on a particular logic.