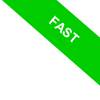
Python any() Function
In Python, there's a built-in function known as any(). This function will return True if, and only if, at least one element within an iterable (think list, set, dictionary, string, and so forth) turns out to be True.
any(oggetto)
The syntax is as simple as: any(object), where 'object' denotes the iterable you're scanning for at least one True element.
Here's what you can expect from the any function:
- True
It will return True if it stumbles upon a True element in the iterable. - False
It will return False if the iterable is barren or devoid of any True elements.
It's worth noting that the any() function is designed to be efficient. It will terminate its search and spit out True the moment it encounters the first True element. So, it doesn't have to necessarily rummage through the entire iterable. This feature is a lifesaver when you're grappling with hefty datasets.
Now, let's turn this theory into practice.
Imagine you have a list containing four Boolean values, like so:
>>> booleans = [False, False, True, False]
You can use the any() function to probe if the list has at least one True value:
>>> any(booleans)
In this case, there's at least one True in our 'booleans' list. So, as expected, any(booleans) gives us True.
True
An interesting observation here is that the any() function stops its search after the third item. It doesn't bother with the fourth item to verify whether it's True or False because it's already found what it was looking for - a True element.
The presence of one True element is enough to pull the plug on the search and return the result without any further delay.
Now, let's create a list devoid of any True values:
>>> booleans = [False, False, False, False]
If we fire up the any() function once again,
>>> any(booleans)
it runs a full scan on all elements of the list, finds no True elements, and thus returns False.
False
Let's shake things up with another example.
Create a list of numbers:
>>> numbers = [0, 1, 2, 3]
Then use the any() function to see if there's at least one True element in it.
>>> any(numbers)
Voila! The any() function returns True. That's because Python treats any number other than zero as True.
True
In this scenario, the function performs two sweeps before yielding the result. It hits the brakes once it encounters the number 1.
Lastly, let's test the any() function on an empty list.
>>> empty = []
Can it find at least one True element in there?
>>> any(empty)
Of course not! The any() function hands out a False in this instance because the list is devoid of elements, to begin with.
False
So, there you have it! The any() function in Python - a handy tool for efficient, targeted searches in your data.