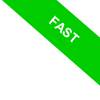
Python's filter() Function
In the vast landscape of Python, the filter()
function stands out as an intrinsic feature. It's designed to sift through an iterable, guided by a function that determines which elements make the cut. Here's the breakdown:
filter(function, iterable)
The function has two primary components:
- Function: A callable that takes an element from the iterable and returns either
True
orFalse
. - Iterable: The collection you're aiming to filter, be it a list, set, string, or any other iterable.
The beauty of filter() lies in its simplicity. If the function deems an element worthy (returns True
), that element finds its way into the output. If not, it's discarded.
However, a nuance to remember is that filter()
doesn't directly return a list. Instead, it gives back a filter object. To see your filtered results in list form, a quick wrap with list()
does the trick.
Let's dive into a practical example.
Imagine you have a list of numbers and you're on a mission to extract only the even ones. Start by crafting a function:
- def is_even(n):
- return n % 2 == 0
With a list like:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9]
Deploy filter()
to do the heavy lifting:
y = filter(is_even, numbers)
Convert the result to a list and display it:
print(list(y))
Voilà! The output reveals the even contenders:
[2, 4, 6, 8]
For those who appreciate brevity, filter() pairs elegantly with lambda functions
y = filter(lambda x: x % 2 == 0, numbers)
print(list(y))
The result remains an ensemble of even numbers:
# [2, 4, 6, 8]
But don't box filter() into just lists. It's versatile.
For instance, to filter strings exceeding a certain length:
phrases = ["hello", "good morning", "hi", "hey"]
y = filter(lambda s: len(s) > 4, phrases)
print(list(y))
The outcome? Words with more than a mere four characters.
['good morning', 'hello']
Or, consider a list of dictionaries. Want to spotlight people over a certain age?
people = [
{"name": "Mary", "age": 30},
{"name": "Luke", "age": 25},
{"name": "Anne", "age": 35},
]
y = filter(lambda p: p["age"] >= 30, people)
print(list(y))
The result is a curated list of those 30 and up:
[{'name': 'Mary', 'age': 30}, {'name': 'Anne', 'age': 35}]
In essence, Python's filter() is more than just a function; it's a testament to the language's power and elegance, enabling sophisticated filtering with minimal fuss.