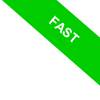
Python's max() Function
In the vast landscape of Python, the max() function stands out as a powerful tool, adept at pinpointing the highest value within iterable objects, such as lists or tuples. Let's break down its syntax:
min(iterable, *[, key, default])
But what if you're dealing with individual values, not nestled within an iterable? No worries. Here's how you tackle that:
min(arg1, arg2, *args[, key])
Invoke the max() function, and it promptly hands you the highest value.
And if faced with duplicate peak values? It simply returns the first one it stumbles upon.
The function's versatility doesn't end there. The key argument lets you introduce a custom comparison function, tailoring the search to your specific needs. Meanwhile, the default argument is your safety net, ensuring you have a fallback return value for empty iterables.
Let's put this into perspective with some examples.
Imagine a list of integers:
numbers = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
max(numeri)
The result? A clear:
9
But the max() function isn't just for lists. It's equally adept at comparing standalone values:
max(4, -2, 7, -5)
And the crown goes to:
7
Strings? max() has got them covered too. It discerns the string positioned later in the alphabetical order.
fruits = ["apple", "banana", "cherry", "orange"]
max(fruits)
The verdict?
orange
For those seeking a more nuanced approach, consider this.
Given a list of tuples symbolizing (x,y) coordinates:
points = [(2, 3), (1, -1), (5, 6), (0, 0)]
To spotlight the point with the loftiest y-component:
max(points, key=lambda p: p[1])
The outcome?
(5, 6)
A word of caution: tread lightly with empty iterables. W
ithout a designated default value, max() won't hesitate to throw an exception.
But, with a touch of foresight and the default argument, you can elegantly sidestep this pitfall.
max([], default="Empty list")
Python graciously offers:
Empty list
This ensures a seamless experience, devoid of unsightly error messages.
In wrapping up, the max() function emerges as an invaluable ally in the quest for the pinnacle value within a dataset.