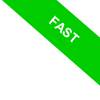
Python's next() Function
Python's next() function lets you fetch the succeeding element from an iterator. The syntax is:
next(object, default)
The function takes two parameters:
- The first parameter (object) is the iterator object. This is mandatory.
- The second parameter (default) is the message that would be displayed in place of a StopIteration exception. This one's optional.
The function retrieves items from the iterator one by one.
If there aren't any more elements, it triggers a StopIteration exception.
Here's a hands-on example.
Let's start by creating an iterator.
>>> my_iterator = iter([1, 2, 3])
Next, fetch the first element.
>>> next(my_iterator)
1
Fetch the second element.
>>> next(my_iterator)
2
Fetch the third element.
>>> next(my_iterator)
2
Now, if you try to fetch an additional element, the next() function raises a StopIteration exception, since there are no more elements left to return.
>>> next(my_iterator)
Traceback (most recent call last):
StopIteration
If desired, you can customize this final message by providing a string as the second argument for the next function.
>>> my_iterator = iter([1, 2, 3])
>>> next(my_iterator, "End" )
1
>>> next(my_iterator, "End" )
2
>>> next(my_iterator, "End" )
3
>>> next(my_iterator, "End" )
End
In this case, Python doesn't raise the StopIteration exception but instead shows the alphanumeric value "End" that you've specified.