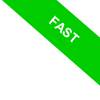
Unpacking Iterables in Python
Python's ability to "unpack" or "destructure" iterables isn't just a neat trick—it's a core feature that epitomizes the language's emphasis on readability and elegance.
So, what's the big deal about unpacking? Simply put, it's a streamlined way to assign values from iterables, such as lists or tuples, to individual variables. Let's dive into the intricacies of this feature and see how it can elevate your Python coding experience.
Consider this: you want to assign elements from a list to separate variables.
With unpacking, you can do this in a single line:
a, b, c = [1, 2, 3]
Here, the list [1,2,3] is effortlessly unpacked, with a
taking the value 1, b
getting 2, and c
receiving 3.
print(a)
1
But the magic of unpacking doesn't end there.
Swapping Variables with Style
Gone are the days of using a temporary variable to swap two variables. With unpacking, it's a breeze:
a, b = b, a
This nifty line swaps the values of a
and b
in a jiffy.
a=1
b=2
a,b=b,a
print(a)
2
Unpacking in Functions
Functions returning multiple values? Unpacking has got you covered:
- def divisione_e_resto(a, b):
- div_int = a // b
- resto = a % b
- return div_int, resto
- div, res = divisione_e_resto(10, 3)
- print(f"Divisione intera: {div}, Resto: {res}")
Here, our function returns both the quotient and remainder of a division. When invoked, unpacking seamlessly assigns these outputs to div and rem.
Unpacking Strings
Strings, being sequences of characters, are ripe for unpacking:
x, y, z = 'abc'
This assigns 'a' to x
, 'b' to y
, and 'c' to z
.
print(x)
a
Unpacking File
Reading a file line by line? Unpacking makes it intuitive:
- with open('file.txt', 'r') as f:
- linea1, linea2, linea3 = f
The variable line1 gets the first line of the file's text, line2 the second, and line3 the third.
Unpacking Dictionaries
While lists and tuples are the usual suspects for unpacking, dictionaries aren't left out.
However, there's a twist: unpacking a dictionary gives you its keys, not the values.
x, y, z = {'nome': 'Giovanni', 'cognome': 'Bianchi', 'eta': 30}
Here, x
gets 'first_name', y
gets 'last_name', and z
gets 'age'.
print(x)
nome
But remember, dictionaries inherently lack order, so unpacking doesn't guarantee a specific sequence.
Extended Unpacking
The asterisk (*) in unpacking is a game-changer. It allows you to assign multiple elements to a single variable:
*x, y = [1, 2, 3, 4]
Here, x
becomes the list [1, 2, 3], while y
snags the value 4.
In essence, y gets the list's last value, while *x gets the list of the remaining elements.
*x, y, z = [1, 2, 3, 4]
In this scenario, Python assigns 4 to z, 3 to y, and the list of remaining elements [1,2] to *x.
Of course, you can place the asterisk * in front of any variable, not just the first one.
x, *y, z = [1, 2, 3, 4]
Now, x and z are assigned the values 1 and 4 respectively, while *y gets the list [2,3].
What happens if the number of labels matches the number of values?
*x, y, z = [1, 2, 3]
If the number of values matches the number of variables, the *x variable gets a list consisting of just one element, i.e., [1].
And if the number of labels isn't enough to accommodate all the elements?
*x, y, z = [1, 2]
If there are no remaining values to assign, the *x variable gets an empty list [].