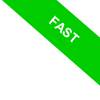
The min() Function in Python
Python's min() function is a versatile tool that effortlessly identifies the smallest element from an iterable, such as a list or tuple, or even when comparing multiple standalone arguments. Consider the following syntax:
min(iterabile, *[, key, default])
or alternatively
min(arg1, arg2, *args[, key])
At its core, the min() function returns the first smallest value it encounters, either within the iterable or among the provided arguments.
In cases where duplicate minimum values exist, it's the first occurrence that gets returned.
The key argument is particularly intriguing. It's a function applied to each item before any comparison takes place. This becomes invaluable when items need to be compared based on specific criteria rather than their inherent values. On the other hand, the default argument is a safety net; it provides a fallback value if the iterable turns out to be empty. Without this and given an empty iterable, min() would raise a ValueError.
Let's delve into some hands-on examples.
Imagine a list of numbers.
numbers = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
To extract the smallest value.
min(numbers)
Python, being the efficient language it is, promptly returns the value 1.
1
But the versatility doesn't end there. The min() function can also discern the smallest value from multiple arguments:
>> min(4, -2, 7, -5)
Here, -5 stands out as the smallest.
-5
When applied to a list of strings, min() defaults to an alphabetical comparison:
fruits = ["apple", "banana", "cherry", "orange"]
min(fruits)
In this scenario, "apple" takes the lead, being alphabetically superior.
orange
The key parameter, however, is where things get even more interesting. It allows for custom comparison logic.
For instance, if you have a list of (x,y) coordinate tuples and aim to pinpoint the one with the lowest y-coordinate:
points = [(2, 3), (1, -1), (5, 6), (0, 0)]
By employing the min() function with a lambda function p: p[1] as the key.
The lambda function takes a tuple p as an argument and returns its second component, i.e., p[1], which represents the y-coordinate of the tuple
min(points, key=lambda p: p[1])
Python returns the tuple (1,-1) based on the y-coordinate.
(1, -1)
Lastly, a word of caution: using min() on an empty object triggers a Python exception.
To elegantly sidestep this, the default parameter is your ally. For instance:
min([], default="Empty list")
Python gracefully returns "Empty list", ensuring your code remains error-free.
Empty list
In essence, the min() function is a testament to Python's power and flexibility, making tasks both simple and complex a breeze.