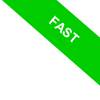
Sorting a List in Python
In this lesson, I will explain how to sort a list in the Python language.
What is sorting a list? Sorting a list means arranging the elements in ascending or descending order. It is an operation that is accomplished through sorting algorithms (or sort).
To sort a list in Python, you can use the sorted() function or the reverse method.
The sorted() function
The sorted() function is one of the most common functions for sorting a list in Python.
sorted(list, reverse=True/False)
The function has two main arguments:
- The name of the list, which is a required parameter.
- The reverse attribute indicates ascending or descending order. It is an optional parameter. If you do not specify it, it performs an ascending sort by default.
The sorted() function returns a new sorted list without modifying the original list.
Here's a practical example.
Here's a practical example:
>>> list = [4, 1, 3, 5, 2]
Use the sorted() function to sort the list in ascending order
>>> list2=sorted(list)
The sorted() function returns a new list with the same elements sorted from smallest to largest.
The result is saved in a second variable called "list2". The elements in list2 are sorted in ascending order.
>>> list2
[1, 2, 3, 4, 5]
Note that the sorted() function does not modify the order of the elements in the original list.
>>> list
[4, 1, 3, 5, 2]
To sort the data in the list in descending order, write the sorted() function with reverse=True as the second parameter.
>>> list2 = sorted(list, reverse=True)
In this case, the sorted() function returns a list with the data sorted in descending order.
>>> list2
[5, 4, 3, 2, 1]
The sort() method
The sort() method is another common method for sorting a list in Python.
lista.sort()
You can use it to sort lists in ascending or descending order.
Note that unlike the sorted() function, the sort() method sorts the original list and does not return a new sorted list.
Here's a practical example.
Create a list with five elements.
list = [4, 1, 3, 5, 2]
Use the sort() method to sort the elements in ascending order.
>>> list.sort()
The method sorts the elements of the list in ascending order.
The result is saved in the "list" variable itself.
>>> list
[1, 2, 3, 4, 5]
Note that the sort() method has modified the order of the elements in the original list.
>>> list
[1, 2, 3, 4, 5]
To sort the list in descending order, use the sort() method on the list and set the optional argument reverse=True
list.sort(reverse=True)
In this case, the sort() method returns the original list sorted in descending order.
>>> list
[5, 4, 3, 2, 1]