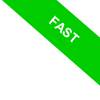
Python's List index() Method
In the world of Python, the index() method can be usefull when you need to find the first occurrence of a certain item within a list. Here's how it works.
list.index(element, start, end)
The method involves three components:
- 'element' is the item you're hunting for in the list
- 'start' is the point in the index where you kick off your search
- 'end' is where you want to wind up your search in the index
The index method will then deliver the position of the item in the list's index.
If the item doesn't show up in the list, the method throws you a curveball and triggers an error message, known as an exception.
Note. The start and end positions are optional parameters. Leave them out, and the index() method will sweep through the entire list for you.
Let's put this into practice.
Imagine you've got a list stored in a variable we'll call "numbers".
>>> numbers = [1, 2, 3, 4, 3, 2, 1, 2, 3]
Then, if you type in numbers.index(3), you're telling Python to look for the first appearance of "3".
>>> numbers.index(3)
Just like that, Python finds "3" chilling at the index position of 2.
2
Even though "3" pops up three times in the list, the index method only gives you the location of its first appearance.
Let's not forget that Python starts its indexing from zero. This means the first item has an index of 0, the second scores 1, the third gets 2, and so on. You'll find the number representing an item's position in the list's index nestled snugly within square brackets.
Now let's try another command.
>>> numbers.index(3,5)
This command tells Python to start the search for "3" from the index position of 5.
In this case, "3" decides to make its first appearance at position 8.
8
And lastly, enter this command
>>> numeri.index(3,5,7)
Here, we're asking Python to look for "3" between the index positions 5 and 7.
However, Python comes up empty this time.
That's because no "3" was found within the chosen range. Consequently, Python raises a red flag and the index() method throws a ValueError exception.
ValueError: 3 is not in list