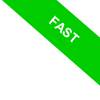
Python's remove() Method
The remove() function in Python offers an efficient way to discard the first instance of a specified value from a list. To be precise, here's the syntax:
list.remove(item)
This method can only be invoked on list-type objects.
The target for removal, referred to as "element", needs to be mentioned in parentheses.
Essentially, this function operates by identifying and removing the first appearance of the specific element within the list.
One important aspect to remember is that this method performs a sequential search. Starting from the first element, it moves systematically towards the last. Should you neglect to specify a value in parentheses, the method will raise an exception, consequently returning an error message.
Let's illustrate this concept with a practical example.
Consider a list composed of four elements.
cities = ['Paris', 'London', 'Rome', 'London']
In this array, 'London' appears twice.
Next, we apply the remove() method, specifying 'London' as the value in parentheses.
cities.remove('London')
Now, let's unveil the updated contents of the list.
print(cities)
You will observe that the method has expunged the first instance of 'London'.
However, the second instance remains undisturbed within the list.
['Paris', 'Rome', 'London']
It's crucial to note that the remove() function modifies the list in-place, without creating a new list. Hence, if your intent is to safeguard the original list, it would be prudent to generate a copy before embarking on the removal operation.