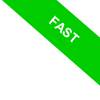
Python's reverse() Method
In Python, there's a handy little method named reverse(). It's a tool to jumble up the order of elements in a list. Simply call it on a list like this:
list.reverse()
In this scenario, think of "list" as any list you've got in your mind to work with.
This trick flips the sequence of items within your list.
But hold on a second. There's an important detail you've got to remember: reverse() doesn't play nice. It goes right ahead and messes with your original list without even creating a new one. So, if you have a list that you don't want changed, make a copy before using this method.
Let's roll up our sleeves and see how this works in action.
First up, let's conjure up a list with a few items.
list = [1, 2, 3, 4, 5]
Right now, this list has got five numeric values.
Next, we'll call on the reverse() method to shake things up.
list.reverse()
Once we've done that, we'll ask the print() function to show us what it's got.
print(list)
Our neat little list [1, 2, 3, 4, 5] has turned topsy-turvy into [5, 4, 3, 2, 1].
[5, 4, 3, 2, 1]
Remember, the reverse() method changes your original list, not caring to create a new one.
If you want to obtain a reversed list without altering the original one, you can use slicing, as demonstrated in the following example:
list = [1, 2, 3, 4, 5]
list2 = list[::-1]
print(list2)
The slicing operation list[::-1] generates a new list with the elements of the original arranged in reverse order.
[5, 4, 3, 2, 1]
And there you have it. The original list is just as you left it, safe and sound.