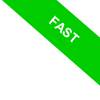
Built-in Objects in Python
In this Python course lesson, I will discuss built-in objects.
What are built-in objects? They are called "built-in" because they are pre-defined objects within a programming language. In general, the built-in objects in the Python language allow you to implement a wide range of applications without the need to manually define each function or data type.
Built-in objects are created by the program when it is executed.
They are stored in the computer's memory as long as the program is running or as long as they are needed.
When they are no longer needed, Python uses a mechanism called garbage collector to free up the memory occupied by the unused objects
What are the built-in objects in the Python language?
The built-in objects in Python are divided into several categories:
- Core data type
These are the fundamental data types in the Python language, such as integers, floats, strings, and booleans. These data types are pre-defined in the Python language and can be used directly without the need to explicitly define them. - Built-in functions
These are the pre-defined functions in Python that can be used directly without the need to define them. Some examples of built-in functions include print(), input(), len(), range(), and many more. - Built-in classes
In Python, builtin classes are inherently part of the language structure. There's no need to go out of your way to define them or import them from external sources - they're readily available for use. Examples of these include classes for integer numbers, often referred to as 'int', those for floating-point numbers, called 'float', and even for lists, known simply as 'list', among others. - Classes
Classes are used in object-oriented programming to define new data types. In Python, you can use classes to create customized objects that can contain data, properties, and related functions called "methods". - Built-in exception types
These are exceptions related to the types of errors that can occur during code execution. In Python, there are several pre-defined exception types, such as TypeError, ValueError, ZeroDivisionError, and more.
The built-in objects in the Python language also include many other features, such as:
The built-in objects of the Python language also include many other features, such as:
- Sequences
These include data structures such as lists, tuples, and strings, which allow you to handle collections of data in an ordered and indexed way. - Sets and dictionaries
Sets are a data structure that allows you to manage sets of data without any ordering. Dictionaries, on the other hand, are a data structure that allows you to manage ordered and indexed data. - Files
These objects allow you to read and write data to files on the hard disk or other input/output devices. - Modules
These are Python language files that contain the definitions of functions, classes, and variables that can be used in programs.
In addition to the built-in objects, Python also has a vast standard library of modules and packages that add additional useful features.
Understanding the built-in objects and standard libraries is essential if you want to learn how to program in the Python language.