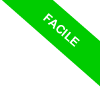
Introspecting Python Objects
In this tutorial, we'll be taking a close look at the concept of introspection in the world of Python programming.
So, why do we need introspection? Essentially, Python's introspection allows us to delve deeper into the internal workings of an object, letting us examine or modify its state, attributes, and kind. Put simply, it's our gateway to understanding what exactly an object is and the functionalities it has up its sleeve.
Python offers a wide array of built-in tools for introspection. Let's explore a few:
- type()
This tool unveils the object's type. For instance, it can be used to discern if an object is a list, a dictionary, an integer, a string, and the list goes on.
Example. Create a list>>> var = [1,2,3,4]
Then check the data type of the variable var using the type() function>>> type(var)
The type function returns the data type. In this case, it's a list<class 'list'>
- dir()
This tool offers a catalogue of attributes and methods tied to the object. This allows us to peek into the different functions an object is capable of calling.
Example. Create a list>>> var = [1,2,3,4]
Then view the list of properties and methods you can call from an object of the "list" type via the dir() function>>> dir(var)
The dir() function displays the list of properties and methods:
['__add__', '__class__', '__class_getitem__', '__contains__', '__delattr__', '__delitem__', '__dir__', '__doc__', ... ecc.
- id()
This tool generates the unique identifier of the object within Python. This identifier becomes particularly useful when we need to check if two references are pointing to the same object.
Example. Create a tuple.
>>> var = (1,2,3,4)
Then display the identifier of the object via the id() function
>>> id(var)
The id() function returns the object's reference in the computer's memory.
140492943321280
- getattr(), setattr(), e delattr()
These tools serve to retrieve, assign, or erase an object's attributes, respectively. - hasattr()
This tool examines if an object possesses a certain attribute. It gives back a boolean value - True if the attribute is present, and False if otherwise.
Example. Create a tuple.>>> var = (1,2,3,4)
Then check if it has the 'real' attribute using the hasattr() function.
hasattr(var,'real')
The function returns False because tuples do not have the 'real' attributeFalse
- in()
This tool is used to determine if a keyword resides within an object.
Example. Create a string in the var variable
>>> var = "Python"
Then check if the substring "th" is found in the string using the in() function.
>>> "th" in var
The function returns True because the substring "th" is indeed found in the string "Python"
True
- is()
This tool checks if two objects share the same reference or identity in the memory.
Example. Create two lists in the variables "list1" and "list2"
>>> list1 = [1,2,3]
Then check if the two objects have the same memory reference using the "is" operator.
>>> list2 = [1,2,3]
>>> list1 is list2
The function returns False because the two lists, although identical, have different memory references. In other words, they are two objects stored at different memory addresses.
False
- isinstance()
This tool comes in handy when we need to verify if an object is an instance of a certain class or a subtype thereof. - issubclass()
This tool checks if a class is a subclass of another.
Having these introspection tools at your disposal can make a world of difference when navigating the depths of Python code.