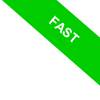
Data Types in Python
In Python, it is not necessary to declare the data type of a variable as the language automatically recognizes it from the value assigned to the variable.
So, what are data types? Data types are categories of data that specify the type of information that can be stored in a variable. For instance, if a variable has an integer data type, it contains an integer value. Similarly, if it has a string data type, then it contains an alphanumeric value. In programming languages, data types are used to create variables and data structures.
Python has several built-in data types, which can be broadly categorized into the following:
data type | description |
---|---|
int | Integer numbers |
float | Floating-point numbers |
complex | Complex numbers |
bool | Boolean values |
str | Strings |
list | Lists |
tuple | Tuples |
range | Ranges |
dict | Dictionaries |
set | Sets |
Here's a practical example.
Assign an integer value to the "year" variable.
>>> year=2020
Note that, in Python, it is not necessary to declare the variable before assignment.
Now, ask Python to show the data type of the "year" variable using the type(year) function:
>>> type(year)
<class 'int'>
Python responds that the "year" variable has the class <class 'int'>, which indicates that it contains an integer number.
Next, assign the value "2020.5" to the "year" variable.
>>> year=2020.5
Python replaces the previous value (2020) with the new value (2020.5) in the variable.
The language automatically changes the data type of the variable from "int" to "float":
>>> type(year)
<class 'float'>
Finally, assign a string of characters to the "name" variable.
>>> name = "Tom"
Then, ask Python to show the data type of the "name" variable using the type(name) function
>>> type(name)
<class 'str'>
Python responds that the variable has the class <class 'str'>, which indicates that it contains a string.
Note that, in other programming languages such as C, it is necessary to declare the data type of the variable before assigning a value. Additionally, it is not possible to change the data type of a variable after declaration