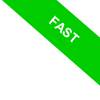
Python Virtual Environments
A virtual environment in Python creates an isolated space where you can install libraries and software packages specific to your project, ensuring there's no impact on the system-wide libraries installed on your machine.
Why use one? Virtual environments allow you to manage dependencies for different projects independently, ensuring that varying library versions don't clash across projects. They're invaluable, particularly when juggling multiple projects with unique dependencies.
Python facilitates the creation and management of these isolated environments through two primary tools: `venv` and `virtualenv`.
Getting Started with `venv`
Available from Python 3.3 onwards, `venv` is a built-in tool that simplifies virtual environment creation.
To set up a virtual environment using `venv`, open your terminal, navigate to your project's directory, and run the following command if you wish to name your environment `myenv`:
python3 -m venv myenv
This command rolls out a `myenv` directory in your current location, equipped with the virtual environment structure.
Inside `myenv`, several automatically generated subdirectories such as `bin` (on macOS/Linux) or `Script` (on Windows), `include`, `lib`, etc., lay the groundwork for an isolated Python runtime.
venv/
├── bin/ # Or "Scripts/" on Windows
│ ├── activate # Script for environment activation (and variants for different shells)
│ ├── pip
│ ├── python
│ └── ...
├── include/
│ └── ... # C headers for extension compilation
├── lib/ # Or "Lib/" on Windows
│ └── python3.8/
│ └── site-packages/
│ └── ... # Installed packages
└── pyvenv.cfg # Environment config file
This setup contains all the essentials for running an isolated Python environment and managing packages within.
- bin/ (on Unix/macOS) or Scripts/ (on Windows)
Houses executables and scripts, including python (or python.exe on Windows), pip, and the activate script. Activating the environment temporarily shifts your shell's PATH to use the Python and pip binaries located here, effectively sandboxing your project. - include/
Contains C headers necessary for compiling Python packages, a feature mainly required on Unix-like systems for C extension compilation. - lib/ (on Unix/macOS) or Lib/ (on Windows)
Stores Python libraries and packages. The lib/ directory further categorizes into subdirectories for each Python version, which contain a site-packages folder where pip installs additional packages. - pyvenv.cfg
A configuration file detailing the virtual environment's setup, including the path to the original Python interpreter.
After creation, you can activate the virtual environment with an OS-specific command.
On Windows, it's as simple as:
myenv\Scripts\activate.bat
For Unix or MacOS, the command changes slightly:
source myenv/bin/activate
Post-activation, your terminal prompt will update to reflect the environment's active status.
Now, any `python` and `pip` commands will run within the confines of your virtual environment, leaving the global Python installation untouched.
To verify the Python version and virtual environment in use, execute which Python on a Linux/macOS system via the command line
which python
or which Python 3 for Windows users.
which python
For example, to install the requests library while within your virtual environment, you'd use pip:
pip install requests
Then, you can proceed to write a script named example.py in the same directory, like so:
import requests
response = requests.get('https://www.wikipedia.org')
print('Status Code:', response.status_code)
Executing this script should output the HTTP response status code to your terminal, typically 200 for a successful request.
Exploring `virtualenv`
As an alternative to `venv`, `virtualenv` provides additional flexibility and backward compatibility with earlier Python versions.
Before using `virtualenv`, you'll need to install it via pip:
pip install virtualenv
Then, creating a new virtual environment is just a matter of:
virtualenv myenv
Activation and deactivation commands for `virtualenv` mirror those of `venv`, tailored to your specific OS.
Deactivating Your Virtual Environment
When your work in the virtual environment is complete, remember to deactivate it using the `deactivate` command.
deactivate
This will revert your shell environment to its default state, allowing you to work with the system-wide Python setup once again.