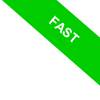
Python Classes
In Python the classes constitute the backbone of object-oriented programming (OOP).
So, what exactly is a class? A class can be seen as a sort of blueprint, a template that delineates the process of creating a certain type of object. Here, an object refers to an aggregation of variables, known in this context as attributes or properties, and functions (or methods), which operate on these variables.
Let's ground this in a practical example.
Python requires the use of the keyword 'class' to define a class.
- class Person:
- age = 25
In this illustration, 'Person' is a basic class featuring a single attribute: 'age'.
It's customary to start a class name with a capital letter.
Contrary to a function, the code inside a class runs instantly when it's defined
An object or an instance of this class can be created using the syntax: Tom = Person().
Tom = Person()
Objects that are created using a class are referred to as instances of that class.
The variable 'Tom' is now an object of the class 'Person'.
You can access the 'age' property of the object by writing 'Tom.age'.
print(Tom.age)
Here, Python retrieves the 'age' property of the 'Tom' object, yielding the value 25.
25
Classes in Python start showing their real potential when we integrate methods into them.
These methods, essentially functions within the class, are then inherited by the objects.
Consider this:
- class Person:
- # example of a property
- age = 25
- # example of a method
- def greet(self):
- return "Hello"
In this code 'greet' is a method attached to the 'Person' class.
This method can be invoked on any instance of the class.
- Mary = Person()
- print(Mary.greet())
Python assigns an object of the 'Person' class to the variable 'Mary'.
The 'greet()' method is subsequently executed, outputting 'Hello'.
Hello
In fact, the 'greet()' method can be invoked on any instance of the 'Person' class, such as 'Mary' and 'Tom'.
All instances or objects of a class inherit the same attributes and methods from that class.
To ascertain whether an object is an instance of a certain class, Python offers the 'isinstance()' function.
For example, check if the 'Mary' object is an instance of the 'Person' class.
isinstance(Mary, Person)
The 'isinstance()' function confirms that the object 'Mary' is indeed an instance of the 'Person' class by returning True.
True
The init method
A special method available to classes is '__init__', where 'init' is encased in two double underscores.
This method is automatically called into action by Python whenever a class instance is created and is generally employed to initialize the object's attributes.
Here's an example:
- class Person:
- # example of an init method
- def __init__(self, name, age):
- self.name = name
- self.age = age
- # example of a method
- def greet(self):
- return f"Hello, my name is {self.name} and I'm {self.age} years old."
When you instantiate the 'Person' class in this case, you need to provide a name and age as parameters to initialize the object's 'name' and 'age' attributes.
It's important to note that passing these parameters is mandatory; failing to do so will lead to an error.
Now, let's create a 'Student' object from the 'Person' class.
Student = Person("Alice", 23)
The 'Student' variable is now assigned an object of the 'Person' class, with the 'name' and 'age' attributes set to 'Alice' and '23', respectively.
Parameters must be separated by commas.
Now, let's invoke the 'greet()' method of the 'Student' object.
print(Student.greet())
This will have Python execute the method, fetch the contents of the 'name' and 'age' attributes, and output the string:
"Hello, my name is Alice and I'm 23 years old."
Special Attributes of a Class
Python's special attributes empower developers with the flexibility to tailor class behavior.
Identifiable by their double underscore prefix and suffix (__name__), these attributes are the keystones for customization.
Take, for example, determining a class's name, which you can do by referencing its __name__ attribute.
class foo():
pass
print(foo.__name__)
foo
Here are a few other class attributes that pack a punch:
- __module__
Shows the module where the class was defined, linking it back to its source. - __init__(self, [...])
The constructor comes into play when a new class instance is born, setting the stage for attribute initialization. - __getattr__(self, name)
This comes handy when you're reaching for an attribute that's playing hide and seek in the object's namespace. - __setattr__(self, name, value)
A gatekeeper for attribute assignments, ensuring every change is intentional. - __del__(self)
The destructor, a courteous nod to cleaning up, is invoked as the object takes its final bow. - __call__(self, [...])
Breathes life into class instances, allowing them to be invoked like a function. - __delattr__(self, name)
Outlines the protocol for attribute deletion, making sure it's a deliberate act.
And the list goes on, with a wealth of special attributes waiting to be discovered and harnessed.
Bear in mind that this tutorial is a basic foray into Python classes.
Classes encompass a lot more, from inheritance and encapsulation to polymorphism—all fundamental pillars of object-oriented programming.
We'll be exploring these in more depth in our forthcoming Python course lessons.