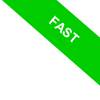
Python Statements
In Python programming, statements play a pivotal role. They are essentially fragments of code that execute specific operations or commands. There are a variety of statements, some of which are simple, and others are compound.
Simple and Compound statements
A simple statement, or 'simple instruction' in Python, is identified by a keyword.
Consider this - assigning a value to a variable is as simple as it gets. Here's an example:
number = 10
Compound statements, conversely, are somewhat more complex. They consist of blocks of code demarcated by the colon symbol ":" which signals the beginning of a code block.
For instance, a 'for' loop encapsulates the concept of a compound instruction, as it requires a block of code:
- for numbers in range(1, 10):
- print(numbers)
Types of Statements in Python
Python showcases an array of statements types, including:
- Assignment statements
These allocate a value to a variable. Here's a simple illustration x = 5. This statement bestows the value 5 onto the variable x.x = 5
- Conditional statements
These allow specific sections of code to be executed when certain conditions are fulfilled. An 'if' statement is a classic example of a conditional instruction.
if x > 0:
print("x is positive") - Loop statements
These facilitate the repeated execution of a code block. 'For' and 'while' loops fall under this category.
for i in range(5):
print(i) - Flow control statements
These govern the progression or 'flow' of a program, facilitating transitions between different code blocks. 'Break', 'continue', and 'pass' exemplify this type of instruction.for i in range(10):
if (i==5): break
print(i) - Function statements
These define a function. For instance:
def say(name):
print("Hello, " + name + "!") - Import statements
These enable the import of modules or functions from other files or modules. For example, importing the math module is as simple as writing - import math.
import math
- Return statements
The 'return' statement concludes the execution of a function and delivers a value.
def addnumbers(a, b):
return a + b
The 'return' statement concludes the execution of a function and delivers a value.
List of Python statements
Finally, let's look at a list of Python statements:
- assert
The assert statement raises an exception if the expression you have specified is false. - del
This statement deletes the reference of an object in memory. - for
This compound statement creates a loop to perform a certain number of iterations. - from
The keyword 'from' allows you to import only certain functions or classes from a module. - if
It's a compound statement that allows you to execute a part of the code only if a particular condition occurs. In other words, it is a conditional structure. - import
This instruction loads into memory the functions and classes of an external module. - pass
The 'pass' statement functions as a placeholder, quite handy when you're in the process of structuring your code. - return
The return keyword terminates a function or method and optionally returns a return value to the calling code. - try except
This compound statement allows you to catch and handle exceptions that may be raised during the execution of a block of code. - with
The 'with' statement enables you to execute a block of code while ensuring that any opened resources are automatically closed at the end. This is especially useful for managing files, databases, and network connections efficiently.