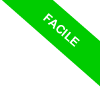
How to use IF ELSE statement in python
In this lesson I'll explain how to use the if else statement in Python language.
The IF ELSE statement is a conditional statement.
if (condition):
conditional code 1
else
conditional code 2
It allows you to perform something only if a particular condition occurs.
How does it work ?
- If the condition is true, the program executes block 1 of code
- If the condition is false, the program executes block 2 of code
Note. The conditional block of statements (block 1 and block 2) must be written a few spaces to the right of the if statement, due to indentation. Code block 1 and 2 can consist of a single statement or multiple statements.
Here is a practical example
hour=12
if (hour<19):
print("it's day")
else:
print("it's not day")
In this code I assign the value 12 to the variable hour.
The if statement checks if the condition (hour <19) is true or false.
- If it is true, it executes print("it's day")
- If it is false, it executes print("it's not day")
Now run the script. The output is as follows:
it's a day
The script prints the message "it's day" to the screen because the condition (hour <19) is true.
Note. The ELSE clause is an additional and optional clause of the if statement. You can also not use it.
I'll give you another practical example.
hour=21
if (hour<19):
print("it's day")
print("end")
In this case the condition (hour <19) is false because hour = 21.
Thus, program execution jumps to the first statement after the IF conditional structure.
The program output is
end
The first useful statement after the IF structure is the print ("end") statement
If everything is clear, try to write an IF ELSE conditional structure and execute it