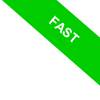
Python print() function
Python's print() function, a built-in feature of the language, is our principal tool for producing output. This function is versatile and can display data on the screen or write it to a file. In terms of usage, it's straightforward:
print(arguments)
The print() function can accept multiple parameters. In the absence of any argument, it simply emits a blank line.
By its default setting, print() directs its output to the standard output, which is typically the console or terminal. However, its flexibility allows for a destination change, which can be a file.
One essential point to keep in mind with Python 3 is the need to always enclose arguments of the print() function within parentheses. This is because print(), in this version, is a function, unlike Python 2 where it's a statement. Ignoring the parentheses will result in a Python error.
Let's walk through a practical example.
Suppose we pass a string as the function's argument.
print("Hello world!")
In this scenario, the print() function receives a string and directs it to the console.
Hello world!
We can also hand over two or more arguments to the print() function, with a comma serving as the delimiter.
print("Hello", "Tom")
Here, the function outputs the arguments separated by a space:
Hello Tom
It's worth noting that the print() function automatically transforms non-string arguments into strings, enabling you to feed it objects of any data type.
print("The answer is", 42)
In this instance, Python takes the number 42, transmutes it into the string "42", and outputs the resultant string.
The answer is 42
The print() function has a built-in feature that creates a new line after each print, which means if you consecutively execute two print() statements, they will appear on separate lines.
print("Hello")
print("world!")
The result of this is printed on two successive lines:
Hello
world!
To keep the printing on the same line, a tweak to the end option of the print() function is necessary. More on that in the next section.
Exploring Advanced Options of print()
print() comes equipped with several advanced settings that you can use to alter its behavior.
print(*objects, sep=' ', end='\n', file=sys.stdout, flush=False)
The variables are:
- *objects: the arguments to print
- sep: the separator character between arguments, defaulting to a space
- end: the character to be printed at the end, a newline (\n) by default
- file: determines where the output should be directed; the console (sys.stdout) is the default destination
- flush: dictates whether the function should empty the buffer right after printing (True) or not (False), default is False
These options open up possibilities for fine-tuning the way your data is printed.
For example, you can define the separator between arguments (a space by default) and stipulate what should be printed at the line's end (a newline character, as standard):
print("This", "is", sep="---", end="*")
print("a test")
In this example, the sep parameter is given "---", so "This" and "is" are printed with "---" in between.
The end parameter is end="*", so the first print() ends with "*", and the subsequent print() continues on the same line.
The output on the screen is:
This---is*a test
By default, the file=sys.stdout option directs output to the standard output, which is your screen.
So, the function print("Hello")
print("Hello")
Is equivalent to the write method of the stdout object:
import sys
sys.stdout.write('Hello'+'\n')
Alternatively, you can specify a physical file "myfile.txt" as the output.
file1 = open('myfile.txt', 'w')
print("Hello", file=file1)
print("world!", file=file1)
In this case, the print function is equivalent to:
file = open('myfile', 'w')
file.write('Hello'+'\n')
Alternatively, to avoid indicating file=file1 in every print() function, you can temporarily redirect standard output (sys.stdout) to write to a file and reset it when you're done. For example:
sys.stdout = open('myfile.txt', 'w')
print("Hello")
print("world!")
sys.stdout.close()
sys.stdout = sys.__stdout__
The first line sys.stdout = open('myfile.log', 'w') directs standard output to write to the physical file "myfile.txt". The following lines print the information to the file. Finally, the file is closed with the close() method. The last line sys.stdout = sys.__stdout__ resets the standard output to its default, which is the screen.
These are just a few practical examples of the powerful capabilities of the print() function.