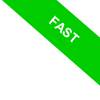
Python return statement
Within Python, a return statement is used to conclude the execution of a function or method, passing a value back to the invoking code.
return x;
Here, x represents the return value.
It's important to note that return is exclusive to the confines of a function or method.
Including a return value is not mandatory. The return command can stand alone to abruptly terminate a function. In such instances, you would simply write "return" without any accompanying value.
return
To bring this to life, let's look at an example.
Consider the function "add_two_numbers" defined in the following snippet:
- def add_two_numbers(num1, num2):
- total = num1 + num2
- return total
- # invoking code
- risult = add_two_numbers(5, 3)
- print(result)
Here, the function takes two parameters, sums them (5+3), and sends the result back using "return total".
This result (which is 8) gets assigned to the variable 'result', which is then printed.
8
If no return value is explicitly indicated, Python will hand back a None type object.
As I touched on earlier, the return keyword can also function without any specific value.
Let's dive into another example:
- def print_message(message):
- print(message)
- return
- # invoking code
- print_message("Hello world!")
This function takes a parameter (message), assigns a string value to it ("Hello world!"), and prints it.
Hello world!
In this instance, the return command wraps up the function, giving control back to the invoking code without supplying a return value.