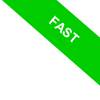
Python's Built-in Classes
Python, inherently, comes with a robust set of built-in classes, always ready to serve any coding need.
So, what does 'built-in' mean? The term 'built-in' signifies that these classes are available without the need for importing external libraries or modules. They are inherent to the Python interpreter itself.
Here are a few examples:
- int
This is the class utilized for representing an integer.x = int(7)
Output
print(x)7
- float
This class is used to represent a floating-point number.x = float(7.3)
Output
print(x)7.3
- str
This class is deployed for representing a string of characters.x = str("Hello, World!")
Output
print(x)Hello, World!
- list
This is the class used to represent a list of elements. A list can contain elements of any type.x = list([1, 2, 3, 4, 5])
Output
print(x)[1, 2, 3, 4, 5]
- tuple
This class is for representing a tuple, which is similar to a list but is immutable.x = tuple((1, 2, 3, 4, 5))
Output
print(x)(1, 2, 3, 4, 5)
- dict
This class is employed for representing a dictionary, which is a collection of key-value pairs.x = dict({"name": "John", "age": 30})
Output
print(x){'name': 'John', 'age': 30}
- set
This class is used to represent a set, which is a collection of unique elements.x = set([1, 2, 3, 1, 2])
Output
print(x){1, 2, 3}
- bool
This class is used to represent a boolean value, which can either be True or False.x = bool(1)
Output
print(x)True
- complex
This is the class for representing a complex number.x = complex(1, 2)
Output
print(x)(1+2j)
- bytes
This class is used to represent a sequence of bytes.x = bytes(5)
Output
print(x)b'\x00\x00\x00\x00\x00'
- bytearray
This class is used to represent a mutable byte sequence.x = bytearray(5)
Output
print(x)bytearray(b'\x00\x00\x00\x00\x00')
- range
This is the class used to represent a sequence of numbers.x = range(5)
Output
print(list(x))[0, 1, 2, 3, 4]
Python doesn't stop here, though. It also includes a variety of other built-in classes, such as object, type, super, etc.
These classes form the cornerstone of Python's object-oriented programming approach, showcasing the language's depth and versatility.