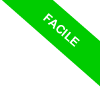
Python's dir() Function
Python's dir() function is an enlightening built-in feature. As if holding a mirror up to any object, it lists all of the attributes and methods that it possesses.
dir(object)
It's always at your disposal since it's part and parcel of Python's comprehensive utility set.
- Invoking dir() without arguments serves as an inventory check of the current local scope, listing all the names available.
- Contrastingly, providing an object as an argument to dir() will result in a detailed listing of that object's attributes and methods.
The beauty of dir() lies in its ability to illuminate the structure of Python objects. It becomes a true lifesaver when you're unsure whether a particular attribute or method is callable by an object.
Here's a quick demonstration
Say we create a string
>>> var = 'Hello world!'
We can then ask dir() to provide an overview of this object
>>> dir(var)
In return, dir() serves a detailed account of all the properties and methods of the string.
Essentially, the dir() function behaves like an index at the back of the documentation on strings.
['__add__', '__class__', '__contains__', '__delattr__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__getnewargs__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__iter__', '__le__', '__len__', '__lt__', '__mod__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__rmod__', '__rmul__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', 'capitalize', 'casefold', 'center', 'count', 'encode', 'endswith', 'expandtabs', 'find', 'format', 'format_map', 'index', 'isalnum', 'isalpha', 'isascii', 'isdecimal', 'isdigit', 'isidentifier', 'islower', 'isnumeric', 'isprintable', 'isspace', 'istitle', 'isupper', 'join', 'ljust', 'lower', 'lstrip', 'maketrans', 'partition', 'removeprefix', 'removesuffix', 'replace', 'rfind', 'rindex', 'rjust', 'rpartition', 'rsplit', 'rstrip', 'split', 'splitlines', 'startswith', 'strip', 'swapcase', 'title', 'translate', 'upper', 'zfill']
This usage of dir() isn't confined to built-in objects. You can harness it to scrutinize any object.
Let's demonstrate this with a class, Person
- class Person:
- def __init__(self, name, age):
- self.name = name
- self.age = age
- def greet(self):
- print(f"Hi, my name is {self.name}")
This class is equipped with two attributes (name, age) and one method (greet).
Now, we instantiate a Person object "p":
p = Person("Mario", 30)
We then pass this object to dir()
print(dir(p))
dir() promptly delivers an output akin to:
['__class__', '__delattr__', '__dict__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__weakref__', 'age', 'name', 'greet']
Interestingly, this includes both the attributes and methods defined in the class (name, age, greet), as well as several special built-in methods, indicated by double underscores __.
These special methods serve a purpose - they allow for the implementation of custom behaviors in Python, such as operator overloading.
Finally, running dir() devoid of any arguments
dir()
Returns an inventory of the local scope, which unsurprisingly includes the Person class we previously defined.
['Person', '__annotations__', '__builtins__', '__cached__', '__doc__', '__file__', '__loader__', '__name__', '__package__', '__spec__', 'p']
In programming terms, "scope" denotes the context where names of variables, functions, and objects are valid within a block of code.