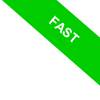
Python's Object Attributes
In today's Python tutorial, we're looking at object attributes.
Each object in Python comes with a set of unique identifiers - we call these "attributes".
Accessing these attributes is a straightforward process: simply write the object's name, add a dot, then the attribute's name, as follows:
object.attribute
Attributes are classified into two categories:
- Methods
These are the 'call-to-action' attributes. You can think of them as your personal helpers, always ready to perform operations when called upon. Always remember to add parentheses after methods, even when you have no arguments to pass. These methods are essentially shared functions that can be invoked and executed on all objects within the same class.
object.method()
- Properties
Now, properties are the quieter attributes. They don't perform tasks, but they give you access to vital object information. Unlike methods, properties don't require parentheses. And yes, all objects of the same type or class possess identical properties.
object.property
Wondering about the difference between methods and properties? Simply put, a method swings into action performing operations on the object, whereas a property is akin to a characteristic value of the object. For instance, if the object is a person, a method might encapsulate an action (like running, walking, or talking), whereas a property might represent the person's age, weight, or height.
Let's delve into a practical example for more clarity.
Assume you've defined a complex number under the variable 'num'
>>> num = 2+3j
It's classified as an object of the "complex" type.
>>> type(num)
<type 'complex'>
The beauty of "complex" type objects is that they come pre-packaged with their own properties and methods.
For instance, try typing num.conjugate()
>>> num.conjugate()
This, is a method – the parentheses are the giveaway.
Even if there are no arguments to pass, never forget to add parentheses to your methods.
The .conjugate() method will promptly return the complex conjugate of the number.
In our example, the conjugate of 2+3j happens to be the complex number 2-3j.
(2-3j)
Now let's test a property. Try typing num.real
>>> num.real
As .real doesn't have parentheses, it's clearly a property.
And it dutifully delivers the real part of the complex number.
In our case, the real part of the complex number num=2+3j is 2.0.
2.0
Refrain from adding parentheses to a property. This will only annoy Python and cause an error. Keep parentheses exclusive to methods, or callable objects.
Complex numbers also have a handy attribute called the .imag property.
Go ahead, type num.imag
>>> num.imag
Here, the .imag property provides the imaginary part of the complex number 2+3j, which happens to be the coefficient 3.0.
3.0
How can you distinguish a callable attribute from a non-callable one? The callable() function comes to the rescue. For instance:
>>> callable(num.conjugate)
This function will return True since the .conjugate attribute is a method applicable to complex type objects. It's definitely not a property.
True
Are you curious to uncover all the attributes hiding in an object?
Well, Python equips you with the dir function to do just that:
dir(oggetto)
Let's put it to the test with dir(num)
>>> dir(num)
And voila! The function generously discloses a list of attributes belonging to 'complex' type objects:
['__abs__', '__add__', '__bool__', '__class__', '__delattr__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getnewargs__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__mul__', '__ne__', '__neg__', '__new__', '__pos__', '__pow__', '__radd__', '__reduce__', '__reduce_ex__', '__repr__', '__rmul__', '__rpow__', '__rsub__', '__rtruediv__', '__setattr__', '__sizeof__', '__str__', '__sub__', '__subclasshook__', '__truediv__', 'conjugate', 'imag', 'real']
And what if you're only interested in checking the existence of a specific attribute within an object?
Python has got you covered with the hasattr() function.
hasattr(oggetto, attributo)
For example, let's probe for the 'conjugate' attribute within num:
>>> hasattr(num,'conjugate')
This function will scrutinize whether the num object possesses the 'conjugate' attribute.
The verdict is True because num is of the 'complex' type, and all objects of this type can readily access the conjugate() method.
True
So, there you have it. With Python, you're always equipped to identify the attributes you can utilize within an object.