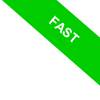
The Python 'if' Statement
Python's 'if' statement is a fundamental tool that executes a section of code based on whether a certain condition is met.
Here's its standard form:
- if condition:
- # code executed if condition is met (True)
We refer to this as a compound statement.
Let's illustrate this with a simple example:
- x = 10
- if x > 5:
- print("x is greater than 5")
In this case, our condition is x > 5.
With x equal to 10, the condition x > 5 is fulfilled.
Thus, the 'if' statement triggers the execution of the enclosed code, outputting "x is greater than 5".
Incorporating 'elif' and 'else' Clauses
The 'if' statement can be expanded using 'elif' and 'else' clauses.
The 'elif' clause is useful for testing additional conditions if the initial condition is not met.
- if condition:
- # code executed if condition is met (True)
- elif condition2:
- # code executed if the first condition is not met (False) and the second condition is met (True)
On the other hand, the 'else' clause allows you to specify a section of code to be executed if none of the preceding conditions are met.
- if condition:
- # code executed if condition is met (True)
- else:
- # code executed if all previous conditions are not met (False)
You can include as many 'elif' clauses as necessary, but an 'else' clause is limited to one per 'if' statement.
- if condition:
- # code executed if condition is met (True)
- elif condition2:
- # code executed if the first condition is not met (False) and the second condition is met (True)
- elif condition3:
- # code executed if the first and second conditions are not met (False) and the third condition is met (True)
- else:
- # code executed if all previous conditions are not met (False)
Note that the 'else' clause is always placed at the end of the 'if' statement.
It's worth noting that 'elif' and 'else' clauses are optional and can be used independently of each other. You may include only 'elif' clauses, only the 'else' clause, neither, or both in an 'if' statement.
Here's an example using both 'elif' and 'else':
- x = 10
- if x > 15:
- print("x is greater than 15")
- elif x > 5:
- print("x is greater than 5 but not greater than 15")
- else:
- print("x is 5 or less")
In this situation, x is assigned a value of 10.
The first condition x > 15 is not fulfilled, causing Python to assess whether the next condition x > 5 is true.
As the condition x > 5 is true, the 'if' statement executes, printing "x is greater than 5 but not greater than 15", then exits the conditional structure.
The 'else' clause is skipped over in this instance since a preceding condition has been satisfied.