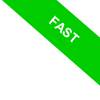
With Statement in Python
The with statement in Python is used to manage the opening and closing of resources like files, network connections, and locks, ensuring that these resources are properly released even in the event of errors. The basic syntax of the `with` statement is:
with expression as variable:
code_block
The expression is typically a function call that returns a context manager object.
This object usually implements the `__enter__` and `__exit__` methods.
- The __enter__ method is automatically executed when the with statement is invoked.
- The __exit__ method is automatically executed at the end of the code block.
Why use the with statement? The `with` statement is a highly useful tool in Python because it simplifies resource management in a safe and clean manner. By using it, you can avoid common mistakes like forgetting to close a file or a connection, thereby enhancing the robustness and readability of your code. It's particularly useful for preventing resource leaks, where resources remain open by mistake.
A Practical Example: File Handling
One of the most common uses of the `with` statement is file handling. Here's an example:
with open('text_file.txt', 'r') as file:
content = file.read()
print(content)
The `with` statement ensures that the file is automatically closed at the end of the code block.
Therefore, there's no need to include a file.close() statement.
This code is equivalent to using a try-except structure where we explicitly close the file by calling `file.close()` in the `finally` block to ensure the file is closed even if an error occurs.
file = open('text_file.txt', 'r')
try:
content = file.read()
print(content)
finally:
file.close()
The __enter__ and __exit__ Methods
In addition to managing predefined resources like files, we can create our own custom context managers by implementing the `__enter__` and `__exit__` methods.
Here's a simple example:
class Foo:
def __enter__(self):
print("Starting resource management")
return self
def __exit__(self, exc_type, exc_value, traceback):
print("Ending resource management")
with Foo():
print("Using the resource")
When we instantiate the class, Python automatically executes the __enter__ method, then runs the code block within the with statement, and finally executes the __exit__ method before exiting.
The output of the program is:
Starting resource management
Using the resource
Ending resource management
In other words, Python automatically executes the __enter__ and __exit__ methods before and after the code block of the with statement, without the need to call them explicitly.
This is particularly useful for managing database connections.
Suppose we want to manage a database connection. We can create a context manager to open and close the connection safely:
class DatabaseConnectionManager:
def __enter__(self):
# Simulating opening the connection
print("Database connection opened")
self.connection = "db_connection"
return self.connection
def __exit__(self, exc_type, exc_value, traceback):
# Simulating closing the connection
print("Database connection closed")
# Using the context manager for the database connection
with DatabaseConnectionManager() as connection:
print(f"Using the {connection}")
In this case, the program's output is:
Database connection opened
Database connection closed
In conclusion, whether you're working with files, network connections, locks, or other resources, the `with` statement is an indispensable tool in your programming toolkit.
The 'as' Clause
The 'as' clause in Python's 'with' statement is used to assign a variable to the object returned by the construct.
with object as variable
This approach makes the code more readable and maintainable, as it allows you to directly access the object with a specific name, simplifying interaction with the object during the execution of the 'with' block.
For example, suppose you want to open a file for reading:
with open('file.txt', 'r') as file:
content = file.read()
print(content)
In this example, the function open('file.txt', 'r') opens the file `file.txt` in read mode (`'r'`).
The 'as' clause assigns the opened file to the variable `file`.
This way, within the 'with' block, you can directly use the `file` variable to read the file's content using the file.read() method, making the code more readable and understandable.