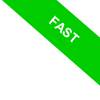
Python del Statement
Python's 'del' statement is your key to eliminating references to objects.
del object
Its functionality spans across various uses - from deleting variables and list elements, to removing keys in a dictionary or object attributes.
Here's how it works in practice.
Variable Removal
Let's say we define a variable "x" with a value of 10 and display its value.
x=10
print(x)
The output, as expected, is 10.
10
We then proceed to delete the variable with 'del'.
del x
Attempting to print the variable's content now results in an error, as Python no longer recognizes "x".
print(x)
Python then throws the following error.
Traceback (most recent call last):
File "/home/main.py", line 1, in <module>
print(x)
NameError: name 'x' is not defined
It's important to note that the 'del' statement only removes the reference to the object and not the object itself.
Therefore, if there are other references to the object, it continues to exist in memory.
For example, consider two labels, "x" and "y", both assigned to the same object: the value 10.
x=y=10
del x
print(y)
Upon deleting "x", Python outputs 10, given that the 'del' statement only severed the link between "x" and the object 10.
The object 10 remains intact, accessible via the "y" label.
Removing a List Item
The 'del' statement's functionality also extends to deleting items from a list.
Take a list stored in a variable "list", for instance.
list = [1, 2, 3, 4, 5]
The list currently has five items: 1, 2, 3, 4, 5.
We then delete the second item (index 1) using 'del'.
del list[1]
Printing the list now results in:
print(list)
Our list is now down to four items.
[1, 3, 4, 5]
Deleting a Dictionary Key
In a similar vein, the 'del' statement can be employed to eliminate a dictionary key.
Consider a dictionary stored in the variable "dictionary".
dictionary = {'a': 1, 'b': 2, 'c': 3}
The dictionary comprises three keys, each paired with a respective value.
We delete the key "b" with 'del'
del dictionary['b']
Then, we print the dictionary.
print(dictionary)
The dictionary now only contains two keys.
{'a': 1, 'c': 3}
In effect, the key "b" is no more.
Deleting a Class or Its Attributes
The del
statement is not just for removing variables; it can also be used to delete an entire class.
Consider a scenario where you define a class and then create several instances of it.
class Example:
class_var = "This is a class attribute"
def __init__(self, instance_var):
self.instance_var = instance_var
example1 = Example("Instance 1")
example2 = Example("Instance 2")
At any point, you can eliminate the class using the del
command.
del Example
What's intriguing is that deleting the class does not wipe out its instances, which will continue to function as usual.
This occurs because the instances clone the class's namespace upon their creation.
Instance attributes, such as "instance_var" in the example above, remain accessible even after the class is deleted. However, class attributes like "class_var" are eradicated along with the class itself, thus they become inaccessible from the instances thereafter.
Deleting Instance Attributes
You can also use the del
statement to remove an instance attribute from an object.
del example1.instance_var
This deletion only affects the specified object, 'example1' in this case, and does not impact other instances such as 'example2', which will continue to hold the attribute 'instance_var'.