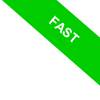
Deleting a Class in Python
This guide provides a straightforward approach to deleting a class in Python using the del statement and explores the effects on instances of that class.
del ClassName
Where "ClassName" refers to the class you intend to remove.
This command eliminates the class from the memory of the session or script.
It's important to note, however, that deleting a class does not immediately impact any instances that have been created from it.
What happens to the class instances? The instances previously created will persist and can still access their instance attributes until they are deleted. Nevertheless, class attributes will be inaccessible to these instances. This behavior has important ramifications for object lifecycle and memory management. The purpose of this guide is to help you navigate managing classes and instances when the classes have been removed but their instances remain active.
Let's walk through a detailed example.
Start by defining a simple class named `Example` with various attributes and methods.
class Example:
class_var = "This is a class attribute"
def __init__(self, instance_var):
self.instance_var = instance_var
def display1(self):
return f"Instance attribute: {self.instance_var}"
def display2(self):
return f"Class attribute: {Example.class_var}"
Proceed by creating several instances of the `Example` class.
example1 = Example("Instance 1")
example2 = Example("Instance 2")
Then, delete the `Example` class using the del command.
del Example
After its deletion, check if the attributes are still accessible through the remaining instances.
Accessing an instance attribute:
Invoking the "display1" method, which uses the instance attribute of "example1", retrieves and displays the value stored in the object.
print(example1.display1())
Instance attribute: Instance 1
This occurs because the existing instances retain a snapshot of the class namespace at their creation, enabling them to function as normal even after the class is removed from the global namespace.
Thus, you can still call methods and attributes on each instance post-deletion.
Attempting to access a class attribute:
Attempting to call the "display2" method, which references the class attribute, in "example1" results in an error.
print(example1.display2())
NameError: name 'Example' is not defined. Did you mean: 'example1'?
Using `Example.class_var` after the class `Example` has been removed results in a `NameError` because the class `Example` no longer exists in the current namespace.
Furthermore, redefining or recreating the class with the same name after its deletion may not replicate the original attributes or behaviors.
Handling errors effectively when accessing a deleted class is crucial. For example, using a try-except structure can help prevent your program from crashing by capturing the `NameError` exception.
try:
print(Example.class_var)
except NameError as e:
print("Error:", e)
In summary, understanding how instances and classes behave when a class is deleted using `del` can significantly aid in effective memory management and predict the operational dynamics in a Python application.