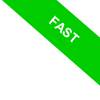
Python's Core Data Types
Let's go through this lesson together, where we'll learn about Python's core data types and how to use them effectively.
So what exactly are these core data types? At their most basic, core data types are the bedrock of the Python programming language. They are intrinsic objects (build-in) that empower you to define variables, assign values, conduct mathematical or logical calculations and so much more. You can even employ these building blocks to create more complex data types.
Python's core data types are categorized into four major groups:
- Numeric Types
- Integer (int)
- Boolean (bool)
- Complex (complex)
- Floating Point (float)
- Set Types
- Sequence Types
- Mapping Types
Each of these data types, whether it's an 'int', 'bool', 'complex', 'float', 'set', 'str', and so on, is commonly referred to as a class. Each class symbolizes a particular type of data.
In Python, these classes can be utilized to construct specific objects, termed instances.
For instance, consider the text "Hello World". This text is an instance of the string class ('str'). Similarly, the number 2020 is an instance of the integer class. In Python parlance, an object of a specific type can also be called an instance of that type. Consequently, whether you call a string an object of the 'str' type or an instance of 'str' type, the implication is identical.
Let's put this theory into practice.
Suppose we assign the value "Hello World" to a variable christened "myVar".
>>> myVar = "Hello World"
If you want to identify the data type of the object 'myVar', deploy the type() function.
>>> type(myVar)
The type() function will return the data type of the object, or in simpler terms, the class you used to construct it.
In this instance, we're dealing with an object of the string type ('str').
<class 'str'>
This technique enables you to authenticate the data type of any object within a Python program.
On a broader level, each object in Python is marked by three distinctive characteristics - its data type, identity, and attributes:
- Data Type
It's the class you tapped into to create the object. For instance, the 'str' class for strings or the 'int' class for integers.
Example. Let's bring this to life with an example. Create an object of the 'int' type:>>> myVar1=2020
Now, let's unearth the data type of this instance using the type() function.>>> type(myVar1)
Thus, this object is an instance of the 'int' type.
<class 'int'> - Identity
Every object in a Python program is assigned a unique identifier.
Example. Let's try this out. Create two objects of the string type:>>> myVar1="Hello World"
Now, let's pull out the identity of the first object using the id() function.
>>> myVar2="Ciao">>> id(myVar1)
Next, reveal the identity of the second object using the same id() function.
140457824779760>>> id(myVar2)
As you can see, each object is tagged with a distinct identity number, setting them apart as unique entities.
140457825312368 - Attributes
Attributes can be thought of as the specific identifiers, properties, and predefined methods associated with objects of a particular data type.
Example. As an example, create an object of the string type:>>> myVar="Hello World"
Then, harness the count() attribute to quantify the instances of the letter "o" in the string.>>> myVar.count("o")
Here, the character "o" graces the string "Hello World" twice.
2
Attributes, with their predefined functions specific to a data type, are a vital tool in the Python programmer's toolkit, saving you the hassle of defining them manually.
This is just another feather in Python's cap, making it the language of choice for many.