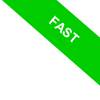
The upper() Method in Python
In this friendly lesson from Nigiara's Python course, we're going to explore the handy upper() method.
So, what's the upper() method all about? Well, it's a nifty little method that lets you convert all the characters in a string to uppercase. Imagine you have a string like "hello world"; the upper method magically turns it into "HELLO WORLD". It's super helpful when you want to compare two strings without worrying about their case - just convert both to uppercase using the upper() method, and you're good to go!
The upper() method comes built-in with string objects, so it's always there when you need it.
To give it a try, just write the name of your string variable, followed by a dot and the upper() method, like this:
string.upper()
Let's walk through a quick example together.
First, create a variable and assign it a string.
>>> string = "this is a sample string"
Next, apply the upper() method to the variable called "string"
>>> string.upper()
Voilà! The method transforms all the letters in the string to uppercase.
THIS IS A SAMPLE STRING
Keep in mind that the upper() method doesn't alter the original string.
So, if you want to hang onto the uppercase version, you'll need to store it in a new variable or overwrite the original one.
>>> uppercase_string = string.upper()
In this case, we've assigned the result to a new variable called "uppercase_string".
The best part is that the upper() method plays well with other string methods, too.
For instance, you can use the upper() method alongside the replace() method.
>>> string = "this is a sample string"
>>> substring="sample"
>>> print(string.replace(substring, substring.upper()))
Here, we've swapped out the substring "sample" in the original string with the same substring in uppercase letters, using both the replace() and upper() methods.
The new, improved string now reads: "this is a SAMPLE string".
this is a SAMPLE string
And there you have it! Happy coding, and have fun experimenting with the upper() method in your Python projects!