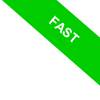
Python's replace() Method
Here's a nifty trick for your Python toolbox: the replace() method. This method is a bit like a search-and-replace function for strings, allowing you to swap out all instances of a specific substring with another one.
string.replace(old_substring, new_substring[, max])
So, what's going on here? This method applies to str objects, with three parameters at play:
- The original substring you want to replace.
- The new substring you'd like to substitute in.
- An optional maximum number (max) of substitutions you'd like to make.
The result? A fresh string, with all instances of the old substring now replaced by your new substring.
And about that third parameter (max) — it's totally optional. If you decide not to specify a number, Python will just replace every single instance of the substring within the string.
Let's see this in action with a simple example.
First, assign this string to a variable named 'text'
text = "My father's father"
Next, use the replace() method to swap the word "father" with "mother".
new_text = text.replace("father", "mother")
It's important to note, your old and new substrings don't need to be the same length.
Finally, you can print the new string using the print() function.
print(new_text)
The output? A shiny new string with all instances of "father" replaced by "mother".
My mother's mother
One thing to bear in mind: the replace() method creates a new string, but it won't alter the content of the original string. If you want to actually change the content of the original string, assign the result of the text.replace() method to the same "text" variable, like this:
text = text.replace("father", "mother")
And what if you want to limit the number of replacements?
Well, you can do that too! By specifying occurrences in the third parameter of the method, you can limit the number of substitutions made.
For instance, if you only wanted to replace the first occurrence of "father" in the string.
new_text = text.replace("father", "mother", 1)
print(new_text)
In this case, the third parameter of the method is 1, meaning it'll only replace the first instance of "father" it finds.
The output? A new string.
My mother's father
In this case, only the first occurrence of the substring "father" got swapped out for "mother".