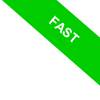
Python's lstrip() Method
Have you ever encountered a bunch of unwanted white spaces at the start of a string in Python? That's where Python's lstrip() method comes into play. This cool little function can efficiently trim off any leading white spaces from a string.
string.lstrip()
This method works seamlessly on String objects.
If you want to get more specific, just pop the character or sequence of characters you'd like to remove into parentheses.
If you don't put anything in, the method will default to banishing those pesky white spaces.
It's good to note that lstrip() creates a fresh string, leaving the original one untouched. The name "lstrip" comes from "left strip", which neatly sums up what it does.
Let's dive into an example.
Suppose we assign a string to the variable 'text', like this:
>>> text = " Hello World!";
Now, if we want to strip the leading white spaces from this string, we just use the lstrip() method.
>>> text.lstrip()
Voila! We get a new string, minus the white spaces at the start.
Hello world!
The original string? Still intact. But we've got a shiny new one without the initial white spaces.
Just a heads-up: lstrip() only deals with the leading white spaces. It doesn't touch any spaces that are tucked within or trailing at the end of the string.
You can also customize lstrip() to specify the character or string of characters you want to remove from the left of the string.
Let's say we have a string with leading asterisks in the variable "bill":
>>> bill = "*****1.100";
By using the lstrip("*") method, we can easily say goodbye to those asterisks:
>>> bill.lstrip("*")
In this case, we've told Python exactly which character we want to eliminate. And just like that, all the "*" characters at the start of the string have vanished.
1.100
So there you have it! You can use lstrip() to remove any character or sequence of characters from the start of a string.