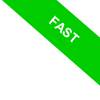
The title() Method for Strings in Python
In this tutorial, I'm going to walk you through the title() method in Python and show you how to use it for manipulating strings.
So, what's the title() method all about? Well, it's a handy function in Python that capitalizes the first letter of each word within a string, while turning the remaining letters into lowercase. This is super useful when you want to format text for titles, making them more visually appealing and easier to read.
In Python, the title() method is specifically designed for String objects.
To use it, simply type:
stringa.title()
Here, "string" represents the variable that holds the character sequence (or text) you want to apply the method to.
If you're new to programming, you might be wondering what a string is. In a nutshell, a string is a sequence of characters enclosed in single or double quotes. For example, "Hello world!" is a string. Strings are used in programming languages to represent and work with text.
Now, let's dive into a practical example.
First, define a String variable called "string":
>>> string = "hello world"
Next, apply the title() method to the content of the variable.
>>> string.title()
Voilà! The result is the same string, but with the initial letters of the words capitalized:
Hello World
As you can see, the title() method has magically transformed "hello world" into "Hello World", capitalizing the first letter of each word.
Note. The title() method in Python can also handle accented letters and special characters without messing up the original string.
Besides capitalizing the first letters of words, the title() method also takes care of converting the other letters in the string to lowercase.
Here's another example. Define this string:
>>> string = "heLLO woRLD"
Now, apply the title() method to the string:
>>> string.title()
The title() method works its magic again, capitalizing the first letters of the words and making all other letters lowercase:
Hello World
So, why would you use the title() method?
Well, it's perfect for creating more readable titles or for comparing two strings.
Take these two strings, for example: "Hello World" and "hello world".
string1 = "Hello World"
string2 = "hello world"
Even though they have different formatting, they actually convey the same information.
By using the title() method, you can transform both strings into "Hello World" and compare them easily.
- string1 = "Hello World"
- string2 = "hello world"
- if string1.title() == string2.title():
- print("The strings are equal")
- else:
- print("The strings are different")
When you run this script, you'll get:
The strings are equal
Thanks to the title() method, you've compared the two strings without worrying about the formatting differences between them.
Just a quick note: if you want to capitalize only the first letter of the string, use the capitalize() method. If you'd like to capitalize all the letters in the string, go for the upper() method.