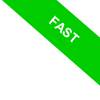
The % Operator in Python
In Python, the % operator is utilized to format strings, akin to the method used in the C programming language.
Let's dive into a practical example.
First, assign an alphanumeric value to the variable "name".
name = "Alice"
Next, use the following syntax to print a string.
print("Hello, %s!" % name)
Here, the symbol %s within the string acts as a placeholder, signifying the position where you wish to insert the value.
Following the string, employ the % operator alongside the value or variable name.
In this scenario, Python will replace the %s placeholder with the value contained in the variable "name."
Hello, Alice!
Strings are immutable objects. Therefore, formatting does not modify the original string but returns a new one.
Understanding Placeholder Formatting
The % operator supports various placeholders, including:
- %c for a single character
- %s for strings. Converts any object using the str() function.
- %r for strings. Converts any object using the repr() function.
- %a for strings. Converts any object using the ascii() function.%s for strings
- %d for integers
- %f or %F for floating-point numbers
- %e for floating point numbers in exponential format (lowercase "e" symbol)
- %E for floating point numbers in exponential format (uppercase "E" symbol)
- %g uses both the exponential (lowercase) format and the floating point decimal format depending on the case
- %G uses both the exponential (uppercase) format and the floating point decimal format depending on the case
- %o for octal numbers
- %x for numbers in base sixteen with lowercase characters
- %X for numbers in base sixteen with uppercase characters
- %% for an actual percent symbol
To illustrate, to format a floating-point value, use %f:
print("This value is %f " % 100)
The %f placeholder is then replaced by the value 100, resulting in:
This value is 100.000000
You can also define the number of digits after the decimal point, as in:
print("This value is %.2f " % 100)
The output now becomes
This value is 100.00
To format an integer display, use %d.
print("This value is %d " % 99.9)
The output is:
This value is 99
Formatting Multiple Variables in a String
To format multiple variables within a single string, enclose the variables in a tuple. For example:
name = "Alice"
age = 25
print("Hello, %s! You are %d years old." % (name, age))
Here, Python replaces the first %s with the variable "name" and the second %d with "age," producing:
"Hello, Alice! You are 25 years old."
It's worth noting that the use of the % operator for string formatting is considered deprecated in Python 3.
Modern best practices favor alternative methods, such as the str.format() method or F-strings.
String Formatting with Keys
In string formatting, a powerful tool at your disposal is the use of keys for integrating dictionary data into strings. This is achieved through the format_map() method.
string.format_map(dictionary)
Let's delve into a hands-on example.
Begin by creating a dictionary. Each key in this dictionary should be an identifier for use within your string. Consider the following:
data = {"name": "Mario", "age": 30}
Here, the variable "data" is a dictionary containing two keys: "name" and "age".
Now, craft the string intended for formatting, embedding the keys from your dictionary within curly braces {}.
string = "My name is {name} and I am {age} years old."
To seamlessly incorporate the dictionary values into your string, apply the .format_map() method.
formatted_string = string.format_map(data)
This technique prompts Python to automatically substitute each key in the string with its corresponding value from the dictionary.
Finally, output the result.
print(formatted_string)
The output will be:
My name is Mario and I am 30 years old.
This approach to string formatting is invaluable when dealing with multiple variables. It allows for effortless updating of values without the need to restructure the string. Moreover, it lends a level of clarity and readability to your code, enhancing its professional quality.
Field Widths in String Formatting
When it comes to string formatting with the % operator, setting the minimum field width is a game-changer for organizing data neatly. It's a simple yet powerful tool for ensuring consistency in your output.
Establishing a fixed minimum width is straightforward: precede the placeholder with the desired number of characters.
- If your data is shorter than the set width, Python will automatically fill in the gap with spaces.
- For data exceeding the width, Python ensures it's fully displayed without any truncation.
For example, to allocate a four-character space for a number, use %4d.
string = "The number is %4d" % 100
print(string)
This approach will print '100' with an extra leading space to meet the four-character requirement.
The number is 100
You can also use zero as a fill character by writing %04d.
string = "The number is %04d" % 100
print(string)
Here, Python outputs 0100.
The number is 0100
Zero as a padding character is effective solely with numeric conversions. In the realm of alphanumeric values, spaces are utilized for padding instead. Consider the following example:
string = "The number is %4s" % '100'
print(string)
In this instance, since '100' is a string, the padding character defaults to a blank space.
The number is 100
When formatting a floating-point number, you have the option to define the number of decimal digits after the point.
For example, to represent the number 100 with ten digits, using zero as a filler and including two decimal digits, you'd use the format %010.2f.
string = "The number is %010.2f" % 100
print(string)
It's important to note that when formatting decimal digits, Python performs rounding of the float number, not truncation.
As a result, the output on the screen will read:
The number is 0000100.00
To left-align the number, simply prepend a minus sign -, using the format %-010.2f.
string = "The number is %-010.2f" % 100
print(string)
Keep in mind, left alignment removes the zero padding while maintaining the specified number of decimal places.
Thus, the final output is left-justified, maintaining two decimal digits:
The number is 100.00
Field width allows for neat columnar arrangement of values, essential for tabulating data.
Consider this script that neatly tabulates squares and cubes of 1, 2, and 3:
- for i in [1, 2, 3]:
- string = "%5d %5d %5d" % (i, i**2, i**3)
- print(string)
The for loop processes the array [1,2,3], calculating and displaying the square and cube for each number in an organized columnar format.
1 1 1
2 4 8
3 9 27
For number sign management, include a space after the % symbol to ensure a blank space precedes positive numbers.
print("The number is % d" % 100)
print("The number is % d" % -100)
This formatting aligns positive and negative numbers in a column.
The number is 100
The number is -100
If you prefer to explicitly display the positive sign, use the + symbol in the format.
print("The number is %+d" % 100)
The number is +100
For more flexibility, opt for a variable width using the wildcard *. This method involves defining the width in a tuple, paired with the data you wish to format.
Here's how it's done:
string = "The name is %*s" % (4, 'Anne')
print(string)
In this example, the tuple sets a minimum of four characters for the first element and provides the data 'Anne' as the second element.
The name is Anne
This method introduces a dynamic element to width management, allowing the minimum size to be adjusted on the fly.
Note: Modern Python versions favor the .format() method or f-strings for string formatting. These newer tools offer enhanced readability and flexibility, making them popular choices among developers.