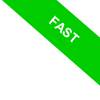
Python's decode() Method
Have you ever wondered how to convert a binary character sequence into a human-readable string in Python? Well, the decode() method comes to the rescue!
Here's how you use it:
string.decode(encoding, error)
This handy method is used for binary objects of the byte type and has two key parameters:
- encoding
This specifies the encoding type to use for decoding the byte sequence. - errors
This guides the method on how to handle decoding errors.
The method then returns a string in the encoding specified in the "encoding" parameter.
You might ask, "What's binary data?" Binary data is a set of numbers that computers use to represent information. Unlike decimal numbers, binary numbers only use two digits: 0 and 1.
Let's dive into a practical example.
First, define binary data in the "data" variable:
>>> data = b"Hello world"
Now, decode the string using the decode() method and specify UTF-8 as the encoding.
>>> data.decode('utf-8')
Voila! The decode() method, with 'utf-8' as the argument, decodes the string into a readable form. You'll get the string "Hello world" in UTF-8 encoding:
Hello world
Keep in mind that the decode() method can convert any binary string into a character string. It's super useful when working with character strings that use various encodings. Just be cautious when handling encoding errors.
Here's another example for you.
Type in this command:
>>> data.decode(encoding="UTF-8", errors="strict")
In this case, you're transforming the information in the data object into a string in UTF-8 format.
With the "errors" parameter, you can indicate how to manage any decoding errors:
- "ignore" means the method will skip undecodable characters.
- "replace" tells the method to use a substitution character instead.
- "strict" raises a UnicodeDecodeError exception if there are invalid bytes in the input sequence. This is the default setting.
Regardless of the choice, the final result remains the same.
Hello world
Dealing with potential decoding errors is crucial when converting a string from one format to another.
So, there you have it—an easy and friendly guide to Python's decode() method. Enjoy decoding!