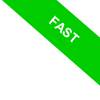
Python's format_map() Method
The format_map() method is a Python powerhouse for string formatting, offering a refined alternative to the traditional string methods.
string.format_map(dictionary)
It operates similarly to the classic format() method, but with a twist: format_map() leverages a single dictionary as its source.
This approach results in a string that's dynamically populated with the dictionary’s contents, providing a streamlined and efficient formatting solution.
The method excels in scenarios involving structured and dynamic data, allowing for seamless integration of data into strings. This reduces coding complexity and boosts readability, a boon for any Python programmer.
Let's unpack format_map() with a concrete example.
Imagine a dictionary containing details of a book:
book = {
"title": "The Adventure of Programming",
"author": "Mario Rossi",
"year": 2023
}
Your goal is to craft a descriptive string about this book.
By choosing format_map() over the standard format(), you can feed the `book` dictionary directly into the method:
description = "The book '{title}' by {author} was published in {year}.".format_map(book)
This snippet neatly substitutes the placeholders with the respective values from the `book` dictionary.
print(description)
The output? A crisp, well-constructed string that eloquently describes the book.
The book 'The Adventure of Programming' by Mario Rossi was published in 2023.
Where format_map() truly shines is in handling dynamic data.
Consider a scenario with multiple books, each represented by a dictionary. You need to generate a description for each one.
Enter format_map(), a champion in a for loop:
- books = [
- {"title": "The Adventure of Programming", "author": "Mario Rossi", "year": 2023},
- {"title": "Python for Everyone", "author": "Luca Bianchi", "year": 2022}
- ]
- for book in books:
- description = "The book '{title}' by {author} was published in {year}.".format_map(book)
- print(description)
Effortlessly, this loop generates a bespoke description for each book, saving you the hassle of individually tailoring arguments for each entry.
The book 'The Adventure of Programming' by Mario Rossi was published in 2023.
The book 'Python for Everyone' by Luca Bianchi was published in 2022.
To wrap up, Python's format_map() is an indispensable string formatting tool, especially potent when dealing with structured data such as dictionaries. Its ability to streamline complex data manipulation into elegant, readable code makes it an essential technique in any Python developer's toolkit.