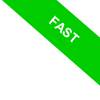
String Formatting in Python
Python offers several techniques for string formatting, each with its unique characteristics. Below are some of the most prevalent methods, along with examples to illustrate their usage:
The Percent Operator (%)
One of the older methods in Python's string formatting toolkit, the percent operator %, harkens back to string formatting in C.
Consider the following example:
name = "Alice"
print("Hello, %s!" % name)
Here, the %s serves as a placeholder for a string or alphanumeric value.
The subsequent % operator directs Python to replace this placeholder with the content of the variable "name."
The resulting output is:
Hello, Alice!
The format() Method
The format() method is a more modern and often more intuitive approach.
Here's how it can be employed:
name = "Alice"
print("Hello, {}!".format(name))
The curly braces within the string are replaced with the value specified within the parentheses of the .format() method.
The outcome is identical:
Hello, Alice!
The format_map() Method
When working with data stored in a dictionary, the format_map() method is an excellent tool.
string.format_map(dictionary)
Consider defining a dictionary with two keys and corresponding values.
data = {"name": "Mario", "age": 30}
Then, craft the string you wish to format.
Incorporate the dictionary keys into the string by placing them inside curly braces.
string = "My name is {name} and I am {age} years old."
Finally, invoke the .format_map() method on the string.
formatted_string = string.format_map(data)
This technique substitutes each key in the string with its matching value from the dictionary.
Printing the string reveals a neatly formatted message using the dictionary's information.
print(formatted_string)
My name is Mario and I am 30 years old.
This method proves invaluable for dynamically formatting data sourced from a dictionary.
F-Strings (Formatted String Literals)
Offering more flexibility, f-strings provide yet another way to format strings.
Here's an illustrative example:
name = "Alice"
print(f"Hello, {name}!")
With f-strings, you initiate the string with an 'f' character before the quotation marks.
Then, use curly braces { } to denote where you want to insert the value or variable. In this case, Python replaces {name} with the content of the variable "name."
The output is:
Hello, Alice!
Whether using the percent operator, the str.format() method, or f-strings, the output is consistently "Hello, Alice!"
Each method has its merits and shortcomings, and the choice of which to use will depend on context, task requirements, and personal preferences.