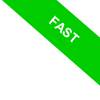
Python bytearray() Function
The bytearray() function in Python is an essential built-in tool, designed to create a sequence of bytes that can be altered or updated.
bytearray()
At their core, bytes are akin to character strings but are employed for binary data representation rather than text.
One of the key features of byte arrays is their mutability; they can be modified post-creation, unlike their byte string (bytes) counterparts, which are fixed and immutable.
Practical Applications: Bytearrays are incredibly handy in scenarios requiring the manipulation of binary data. Whether it's for file operations, network communication, or other activities needing a changeable binary data format, bytearray proves to be a versatile and effective solution.
Creating a bytearray
Python offers several ways to create a bytearray: starting with an empty one, using a string, an iterable of integers, or even a bytes object.
For example, an empty bytearray is simply created by calling the function without any arguments.
ba=bytearray()
After its creation, you can append elements using the extend() method:
ba.extend(b'hello')
The 'b' prefix here denotes a byte string.
Printing the bytearray reveals its nature:
print(ba)
bytearray(b'hello')
Notice it's not just a string; it's a bytearray, where each element represents a number ranging from 0 to 255.
Alternatively, you can initialize a bytearray with specific elements right away, bypassing the extend method. For instance:
ba=bytearray(b'hello')
This approach yields the same result.
print(ba)
bytearray(b'hello')
Accessing Bytearray Elements: Bytearrays allow individual access to their elements through slicing and indexing.
Accessing an element returns an integer (int), not the character itself. This is because the data is stored as numeric bytes.
print(ba[0])
104
This number, 104, corresponds to the Unicode/ASCII value of 'h', the first character in 'hello'.
print(chr(104))
h
Printing the bytearray element by element reveals a sequence of numbers, each between 0 and 255.
- for i in ba:
- print(i)
104
101
108
108
111
These are the codes for 'h', 'e', 'l', and 'o', respectively.
Creating Bytearrays from Integers: It's also possible to create a bytearray using an iterable of integers, each representing a byte (from 0 to 255).
For instance, to represent the string 'hello' in bytes, you can use its ASCII codes in a list:
ba = bytearray([104, 101, 108, 108, 111])
And the output remains consistent.
print(ba)
bytearray(b'hello')
You can also create a bytearray from a string, but you'll need to specify the encoding, like UTF-8.
ba = bytearray('hello', 'UTF-8')
This method also produces a familiar result.
Bytearray Manipulation
Manipulating bytearrays in Python allows for a range of operations, akin to those you'd perform on lists. This includes adding, removing, or altering elements.
Bytearrays come equipped with methods found in byte strings and mutable sequences. These include append, insert, remove, pop, replace, and more.
Let's begin by defining a bytearray object to explore these functionalities.
ba=bytearray(b'hello')
1] Adding Elements
To append new elements, the extend() method seamlessly adds them to the existing bytearray.
ba.extend(b'World')
This appends the new byte string to the existing one.
print(ba)
bytearray(b'helloWorld')
For single-element additions, append() is the go-to method.
ba.append(33)
Here, 33 represents the Unicode code for '!'
The bytearray now includes an additional element.
print(ba)
bytearray(b'helloWorld!')
2] Modifying Elements
Altering individual elements in a bytearray is straightforward. For example, changing 'h' to an uppercase 'H' is as simple as:
ba[0] = ord('H')
Printing the bytearray now reflects this change.
print(ba)
bytearray(b'HelloWorld!')
3] Removing Elements
Removing elements can be achieved using either the remove() or pop() methods. The remove() method targets the first occurrence of a specified value, whereas pop() eliminates an element at a given index or the last one by default.
For instance, using remove(33) will get rid of the first byte valued 33.
ba.remove(33)
This action deletes the exclamation mark.
print(ba)
bytearray(b'HelloWorld')
On the other hand, pop(0) removes the byte at the zero index.
ba.pop(0)
This leads to the removal of the letter 'H'.
print(ba)
bytearray(b'elloWorld')
4] Inserting Elements
Inserting an element at a specific index is effortlessly done with the insert() method.
ba.insert(0,104)
This action places '104', corresponding to 'h', at the zero index, restoring the bytearray to its original form.
print(ba)
bytearray(b'helloWorld')
4] Concatenating Bytearrays
Bytearrays can be concatenated using the + operator, creating a unified bytearray.
ba1 = bytearray(b'hello')
ba2 = bytearray(b'World')
ba=ba1+ba2
This results in a combined bytearray.
print(ba)
bytearray(b'helloWorld')
When a bytearray is concatenated with a byte object, the resultant type is that of the first operand. In this case, since the first operand is a bytearray, the result remains a bytearray.
ba1 = bytearray(b'hello')
byte2 =b'World'
ba=ba1+byte2
print(ba)
bytearray(b'helloWorld')
In a different scenario, concatenating a byte object with a bytearray results in a byte object, as illustrated here .
byte1 = b'hello'
ba2=bytearray(b'World')
ba=byte1+ba2
print(ba)
b'helloWorld'
These are some of the essential operations you can apply to bytearrays in Python, demonstrating their versatility and ease of use.