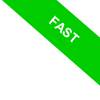
Python's isspace() Method
Have you ever wondered about Python's isspace() method? It's a handy little tool that allows you to check whether a string is made up entirely of whitespace characters or not. Here's how it works:
string.isspace()
Pretty straightforward, right? This method is only for String objects.
If your string consists only of whitespace characters, the isspace() method will return true. However, if there are any other characters present - that is, anything that's not a whitespace character - it will return false.
What exactly are these whitespace characters? They are simply the characters in a string that don't produce a visible mark but serve to create space within the text. Common examples are spaces, tabs (/t), and line breaks (/n). They may not be visible when you print out a string's contents, but they're crucial for formatting and text organization. So when you need to check if a string is empty or just filled with these whitespace characters, the isspace() method is the tool you'd want to use.
Let's walk through a real example together.
Imagine you have a string variable, something like this:
string = "
"
Now, let's put our isspace() method to the test. Does this variable only contain whitespace characters?
string.isspace()
And there you have it! It returns True, because our string, while appearing empty, is actually holding a space
True
Let's try another example, just for kicks.
This time, we'll define an empty string.
>>> string = ""
And let's ask our trusty isspace() method again if it contains any whitespace characters.
>>> string.isspace()
This time, the result is False. Why? Because our string is genuinely empty, no spaces hiding here!
False
Note. Just a heads up though, isspace() only recognizes the standard whitespace characters defined by Unicode. So if there are any custom or non-standard spaces lurking in your string, isspace() won't be able to spot them.
Hope that helps! Python is a fun language to explore, and it's little methods like isspace() that make it so versatile and user-friendly. Happy coding!