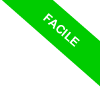
Python's format() Method
Let's explore the format() method in Python, a versatile tool that greatly enhances the readability and flexibility of string formatting.
str.format(x)
In this context, "str" refers to a string containing placeholders, marked by curly braces {}.
The parameter "x" is one or more values you intend to inject into the string, separated by commas.
Essentially, the format() method replaces the {} placeholders within the string with the x values you specify.
Why is it important? This method is fundamental for crafting formatted strings in Python. It allows you to build a string with certain parts fixed while others dynamically adapt based on your input. Think of it as a string template that's both stable and adaptable, letting you insert and tweak data without disrupting the overall format.
An Illustrative Example
Begin by defining a string and incorporating a placeholder {}.
greeting = "Hello, {}!"
Next, apply the format() method to the string, inserting the word "world" in the parentheses.
print(greeting.format("world"))
By doing so, the method replaces the {} placeholder with "world".
The final output appears as:
Hello, world!
Index-Based Formatting
The format() method also allows the use of indices within the curly braces to dictate the order of values.
For example, create a sentence with two numbered placeholders {0} and {1}.
sentence = "{1} and {0} are renowned scientists."
Invoke the format() method with two distinct arguments, each a string separated by a comma.
print(sentence.format("Feynman", "Einstein"))
In this instance, Python assigns "Feynman" to the first index {0} and "Einstein" to the second index {1}.
The resulting output is:
Einstein and Feynman are renowned scientists.
Notice how 'Einstein' precedes 'Feynman', despite being the second argument. This is because, within the string, the placement of index {1} comes before index {0}.
Here, the display sequence is governed by the indices' positions within the curly braces, not the order in which arguments are passed.
Keyword Formatting
Enhance code clarity by employing keyword placeholders.
Consider this example:
sentence = "{name} received the Nobel Prize in {field}."
Each placeholder is labeled for clarity: {name} and {field}.
Then, invoke the format() method, passing the labels "name" and "field" with the corresponding values "Feynman" and "physics".
print(sentence.format(name="Feynman", field="physics"))
The method matches "Feynman" and "physics" to the {name} and {field} tags respectively.
As a result, you get:
Feynman received the Nobel Prize in physics.
Here, the arrangement is determined by the placement of labels within the braces, independent of the argument order.
Keywords can be mixed with indices or generic placeholders for added flexibility.
sentence = "{0} received the Nobel Prize in {field}."
print(sentence.format("Feynman", field="physics"))
Feynman received the Nobel Prize in physics.
Important: Assign non-keyword values before keyworded ones to avoid errors.
For instance, in the following example, the non-keyword value "physics" should precede the keyworded name="Feynman".
sentence = "{name} received the Nobel Prize in {}."
print(sentence.format("physics",name="Feynman))
Feynman received the Nobel Prize in physics.
Mastering Dictionary Value Formatting
Discover how the format() method elegantly formats strings using dictionary keys. Explore this technique here.
Imagine defining a dictionary like this:
info_scientist = {
"name": "Richard Feynman",
"field": "Physics",
}
Now, let's craft a sentence that leverages this information using str.format(), directly referencing the dictionary keys.
sentence = "{name} won the Nobel Prize in {field}"
print(sentence.format(**info_scientist))
The ** operator before "info_scientist" efficiently unpacks the dictionary.
Each key-value pair is seamlessly passed as an individual argument to the format() function.
The output elegantly displays:
Feynman won the Nobel Prize in Physics.
This technique simplifies incorporating dictionary data into formatted strings in Python with ease and precision.
Sequencing Your Formatting
Formatting sequences is just as intuitive.
Start by defining a list or a tuple.
string='{1} employs {0[1]}'
output=string.format(('C++', 'Python'), 'Tom')
print(output)
This script smartly uses the format() method, embedding tuple values into placeholders.
For instance, {0[1]} refers to the tuple's second item, "Python," as indexed by [1].
Tom employs Python
A quick note: avoid negative indices like [-1] to prevent errors.
Numeric Formatting with Precision
The str.format() method is exceptionally versatile, especially for numeric formatting.
It's ideal for controlling decimal precision, for instance.
number = "Pi is roughly {0:.2f}"
Here, {0:.2f} specifies a floating-point number rounded to two decimal places.
Invoke format() with the value 3.14159:
print(number.format(3.14159))
It neatly rounds 3.14159 to 3.14.
Thus, the output reads:
Pi is roughly 3.14
Text Alignment and Spacing: A Study in Elegance
The format() method also provides sophisticated tools for text alignment and spacing.
Consider this string example:
text = "| {:<10} | {:^10} | {:>10} |"
Here, "<" left-aligns, "^" centers, and ">" right-aligns the text. The "10" indicates a ten-character field.
Execute format() with "Rome", "Paris", and "London" as arguments:
print(text.format("Rome", "Paris", "London"))
"Rome" aligns left, "Paris" centers, and "London" aligns right, demonstrating:
|Rome | Paris | London|
This method enhances the neatness and readability of your code, offering a professional touch to string formatting.